使用Java实现并给出xml配置:应用程序一般允许用户自己定义配置信息,如界面背景颜色、字体大小和字体颜色等,尝试使用SharedPreferences保存用户的自定义配置信息,并在程序启动时自动加载这些自定义的配置信息。
时间: 2024-03-03 18:50:21 浏览: 26
好的,下面是Java实现并给出xml配置的代码:
1. 在res目录下创建一个xml文件,例如:preferences.xml
```xml
<?xml version="1.0" encoding="utf-8"?>
<PreferenceScreen xmlns:android="http://schemas.android.com/apk/res/android">
<PreferenceCategory android:title="用户配置">
<EditTextPreference
android:key="background_color"
android:title="背景颜色"
android:summary="设置界面背景颜色"
android:defaultValue="#ffffff" />
<EditTextPreference
android:key="font_size"
android:title="字体大小"
android:summary="设置字体大小"
android:defaultValue="16" />
<EditTextPreference
android:key="font_color"
android:title="字体颜色"
android:summary="设置字体颜色"
android:defaultValue="#000000" />
</PreferenceCategory>
</PreferenceScreen>
```
2. 在Java代码中获取和保存用户配置信息
```java
public class MainActivity extends AppCompatActivity implements SharedPreferences.OnSharedPreferenceChangeListener {
private SharedPreferences sharedPreferences;
private View layout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 获取用户配置信息
sharedPreferences = PreferenceManager.getDefaultSharedPreferences(this);
String backgroundColor = sharedPreferences.getString("background_color", "#ffffff");
int fontSize = Integer.parseInt(sharedPreferences.getString("font_size", "16"));
String fontColor = sharedPreferences.getString("font_color", "#000000");
// 设置界面背景颜色
layout = findViewById(R.id.layout);
layout.setBackgroundColor(Color.parseColor(backgroundColor));
// 设置字体大小和颜色
TextView textView = findViewById(R.id.text_view);
textView.setTextSize(fontSize);
textView.setTextColor(Color.parseColor(fontColor));
// 监听用户配置信息的变化
sharedPreferences.registerOnSharedPreferenceChangeListener(this);
}
@Override
public void onSharedPreferenceChanged(SharedPreferences sharedPreferences, String key) {
// 获取用户修改的配置信息
String backgroundColor = sharedPreferences.getString("background_color", "#ffffff");
int fontSize = Integer.parseInt(sharedPreferences.getString("font_size", "16"));
String fontColor = sharedPreferences.getString("font_color", "#000000");
// 更新界面背景颜色
layout.setBackgroundColor(Color.parseColor(backgroundColor));
// 更新字体大小和颜色
TextView textView = findViewById(R.id.text_view);
textView.setTextSize(fontSize);
textView.setTextColor(Color.parseColor(fontColor));
}
@Override
protected void onDestroy() {
super.onDestroy();
// 停止监听用户配置信息的变化
sharedPreferences.unregisterOnSharedPreferenceChangeListener(this);
}
}
```
3. 在AndroidManifest.xml文件中添加PreferenceActivity的声明
```xml
<activity android:name=".SettingsActivity" />
```
4. 创建一个SettingsActivity类来显示用户配置信息
```java
public class SettingsActivity extends PreferenceActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
addPreferencesFromResource(R.xml.preferences);
}
}
```
5. 在MainActivity中添加一个菜单项来跳转到SettingsActivity
```java
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.action_settings:
Intent intent = new Intent(this, SettingsActivity.class);
startActivity(intent);
return true;
default:
return super.onOptionsItemSelected(item);
}
}
```
6. 在res目录下创建一个menu文件夹,在其中创建一个main.xml文件来添加菜单项
```xml
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+id/action_settings"
android:title="设置"
android:orderInCategory="100"
android:showAsAction="ifRoom" />
</menu>
```
以上就是使用SharedPreferences保存用户的自定义配置信息,并在程序启动时自动加载这些自定义的配置信息的Java实现并给出xml配置。
相关推荐
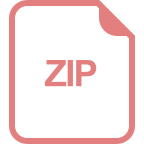
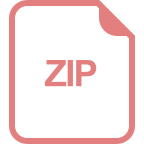
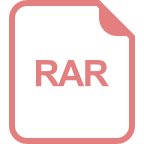














