Qtextedit边沿触发
时间: 2023-08-02 17:06:22 浏览: 87
如果您正在使用Qt中的QTextEdit,那么可以使用QTextCursor来检测文本编辑器中的边沿触发事件。以下是一个示例:
```python
from PyQt5.QtWidgets import QTextEdit, QApplication
class MyTextEdit(QTextEdit):
def __init__(self, parent=None):
super(MyTextEdit, self).__init__(parent)
self.setVerticalScrollBarPolicy(Qt.ScrollBarAlwaysOff)
self.setHorizontalScrollBarPolicy(Qt.ScrollBarAlwaysOff)
self.setLineWrapMode(QTextEdit.NoWrap)
def event(self, event):
if event.type() == QEvent.MouseMove:
cursor = self.cursorForPosition(event.pos())
if cursor.atStart() or cursor.atEnd():
QApplication.setOverrideCursor(Qt.ArrowCursor)
return True
return super(MyTextEdit, self).event(event)
def mousePressEvent(self, event):
if event.button() == Qt.LeftButton:
cursor = self.cursorForPosition(event.pos())
if cursor.atStart():
self.setTextCursor(cursor)
QApplication.setOverrideCursor(Qt.SizeHorCursor)
self._drag_start_x = event.pos().x()
return
elif cursor.atEnd():
self.setTextCursor(cursor)
QApplication.setOverrideCursor(Qt.SizeHorCursor)
self._drag_start_x = event.pos().x()
return
super(MyTextEdit, self).mousePressEvent(event)
def mouseMoveEvent(self, event):
if event.buttons() & Qt.LeftButton:
diff = event.pos().x() - self._drag_start_x
cursor = self.textCursor()
if cursor.atStart():
if diff < 0:
return
cursor.movePosition(QTextCursor.Left, QTextCursor.KeepAnchor)
elif cursor.atEnd():
if diff > 0:
return
cursor.movePosition(QTextCursor.Right, QTextCursor.KeepAnchor)
else:
return
self.setTextCursor(cursor)
self._drag_start_x = event.pos().x()
else:
super(MyTextEdit, self).mouseMoveEvent(event)
def mouseReleaseEvent(self, event):
if event.button() == Qt.LeftButton:
QApplication.restoreOverrideCursor()
super(MyTextEdit, self).mouseReleaseEvent(event)
```
这段代码会在鼠标移动到文本编辑器的边缘时显示一个箭头光标,并且在鼠标按下并拖动边缘时,会使文本编辑器的宽度相应地增加或减少。
阅读全文
相关推荐
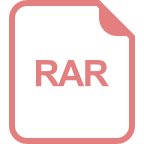
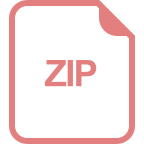
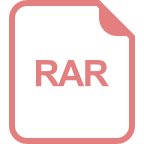














