自行选取图片数据集,例如minist数据集,任意选取神经网络算法,编程实现手写体识别。
时间: 2024-12-11 17:19:12 浏览: 9
手写数字识别通常是通过深度学习中的卷积神经网络(Convolutional Neural Networks, CNN)来完成的,比如经典的MNIST数据集就是一个常用于入门级机器学习教程的简单示例。MNIST包含了60,000张训练图片和10,000张测试图片,每张图片都是28x28像素的手写数字。
以下是使用Python和Keras库(基于TensorFlow)的一个简化的步骤:
1. 导入所需的库:
```python
import keras
from keras.datasets import mnist
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
```
2. 加载并预处理数据:
```python
(x_train, y_train), (x_test, y_test) = mnist.load_data()
x_train = x_train.reshape(-1, 28, 28, 1) / 255.0 # 归一化像素值
x_test = x_test.reshape(-1, 28, 28, 1) / 255.0
y_train = keras.utils.to_categorical(y_train)
y_test = keras.utils.to_categorical(y_test)
```
3. 构建CNN模型:
```python
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dense(10, activation='softmax') # 输出层有10个节点,对应0-9的分类
])
```
4. 编译和训练模型:
```python
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(x_train, y_train, epochs=10, validation_data=(x_test, y_test))
```
5. 测试模型性能:
```python
test_loss, test_acc = model.evaluate(x_test, y_test)
print(f"Test accuracy: {test_acc}")
```
阅读全文
相关推荐
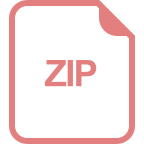
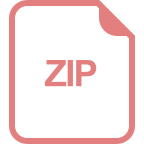
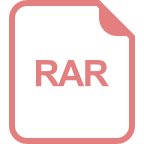
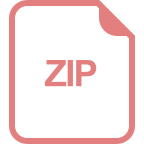
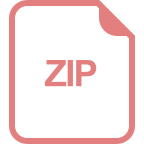
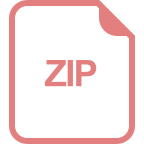
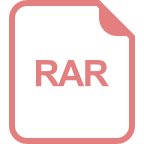
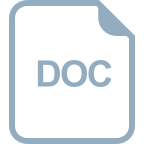
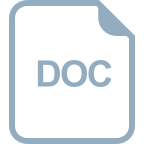







