ndk TensorFlow example code
时间: 2024-05-10 19:15:21 浏览: 77
Here is an example of using TensorFlow with NDK in Android:
1. First, you need to download the TensorFlow library for Android from the official website. Then, extract the archive and copy the "libtensorflow_inference.so" file to your project's jniLibs folder.
2. Create a new C++ file in your project's jni folder, for example "tensorflow_jni.cpp". This file will contain the code that interacts with the TensorFlow API.
3. Include the TensorFlow header files in your C++ file:
```c++
#include <jni.h>
#include <string>
#include "tensorflow/core/public/session.h"
#include "tensorflow/core/platform/env.h"
```
4. Define a function that loads the TensorFlow model:
```c++
JNIEXPORT jlong JNICALL
Java_com_example_tensorflow_TensorFlowModel_loadModel(JNIEnv *env, jobject thiz, jstring model_path) {
const char *path = env->GetStringUTFChars(model_path, 0);
tensorflow::Session *session;
tensorflow::SessionOptions options;
tensorflow::Status status = tensorflow::NewSession(options, &session);
if (!status.ok()) {
// error handling
}
tensorflow::GraphDef graph_def;
status = tensorflow::ReadBinaryProto(tensorflow::Env::Default(), path, &graph_def);
if (!status.ok()) {
// error handling
}
status = session->Create(graph_def);
if (!status.ok()) {
// error handling
}
env->ReleaseStringUTFChars(model_path, path);
return reinterpret_cast<jlong>(session);
}
```
This function takes the path to the TensorFlow model file as input, loads it into a session and returns a pointer to the session object.
5. Define a function that runs the TensorFlow model:
```c++
JNIEXPORT jfloatArray JNICALL
Java_com_example_tensorflow_TensorFlowModel_runModel(JNIEnv *env, jobject thiz, jlong session_ptr, jfloatArray input_data) {
tensorflow::Session *session = reinterpret_cast<tensorflow::Session *>(session_ptr);
jfloat *input = env->GetFloatArrayElements(input_data, NULL);
const int input_size = env->GetArrayLength(input_data);
tensorflow::Tensor input_tensor(tensorflow::DT_FLOAT, tensorflow::TensorShape({1, input_size}));
float *input_tensor_data = input_tensor.flat<float>().data();
memcpy(input_tensor_data, input, input_size * sizeof(float));
std::vector<tensorflow::Tensor> output_tensors;
tensorflow::Status status = session->Run({{"input", input_tensor}}, {"output"}, {}, &output_tensors);
if (!status.ok()) {
// error handling
}
const int output_size = output_tensors[0].shape().dim_size(1);
jfloatArray output_data = env->NewFloatArray(output_size);
env->SetFloatArrayRegion(output_data, 0, output_size, output_tensors[0].flat<float>().data());
env->ReleaseFloatArrayElements(input_data, input, JNI_ABORT);
return output_data;
}
```
This function takes the session pointer and the input data as input, runs the session with the input data and returns the output data as a float array.
6. Finally, define the JNI interface functions in your "Android.mk" file:
```makefile
LOCAL_PATH := $(call my-dir)
include $(CLEAR_VARS)
LOCAL_MODULE := tensorflow_jni
LOCAL_SRC_FILES := tensorflow_jni.cpp
LOCAL_C_INCLUDES := $(LOCAL_PATH)/../include
LOCAL_LDLIBS := -llog -ljnigraphics -landroid
LOCAL_LDFLAGS += -L$(LOCAL_PATH)/../libs/$(TARGET_ARCH_ABI) -ltensorflow_inference
include $(BUILD_SHARED_LIBRARY)
```
That's it! You can now call the "loadModel" and "runModel" functions from your Java code to use TensorFlow in your Android app.
阅读全文
相关推荐
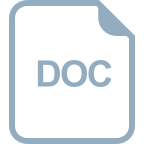
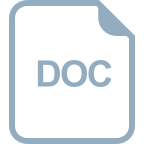
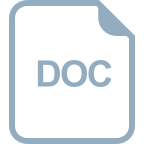

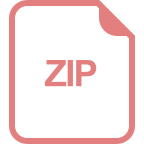
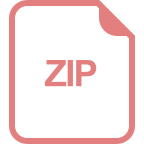
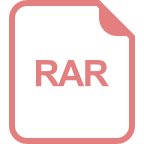
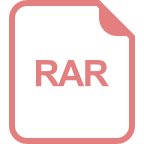
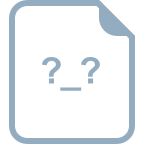
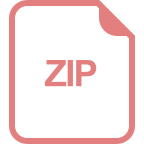
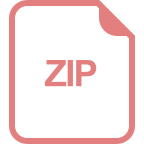
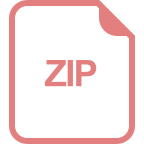
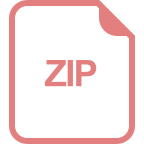
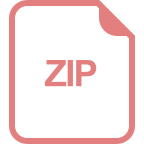
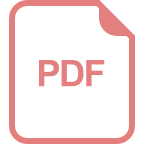
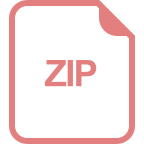
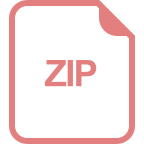
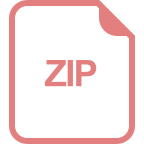
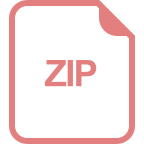