用C语言编写一段短程序代码包含列表,堆栈,队列
时间: 2023-05-28 10:07:00 浏览: 94
以下是一个简单的示例程序,包含了列表、堆栈和队列的实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 列表
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
// 堆栈
void push(Node** top, int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = *top;
*top = newNode;
}
void pop(Node** top) {
if (*top == NULL) {
printf("Stack is empty.\n");
return;
}
Node* nodeToRemove = *top;
*top = (*top)->next;
free(nodeToRemove);
}
// 队列
void enqueue(Node** front, Node** rear, int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
if (*front == NULL) {
*front = newNode;
} else {
(*rear)->next = newNode;
}
*rear = newNode;
}
void dequeue(Node** front, Node** rear) {
if (*front == NULL) {
printf("Queue is empty.\n");
return;
}
Node* nodeToRemove = *front;
*front = (*front)->next;
free(nodeToRemove);
if (*front == NULL) {
*rear == NULL;
}
}
int main() {
// 创建一个列表
Node* head = (Node*)malloc(sizeof(Node));
head->data = 1;
head->next = NULL;
Node* node2 = (Node*)malloc(sizeof(Node));
node2->data = 2;
node2->next = NULL;
Node* node3 = (Node*)malloc(sizeof(Node));
node3->data = 3;
node3->next = NULL;
head->next = node2;
node2->next = node3;
// 打印列表
printf("List: ");
printList(head);
// 创建一个堆栈
Node* top = NULL;
// 压入堆栈中的元素
printf("Pushing 1, 2, 3 onto the stack...\n");
push(&top, 1);
push(&top, 2);
push(&top, 3);
// 弹出堆栈中的元素
printf("Popping from the stack...\n");
pop(&top);
pop(&top);
pop(&top);
// 创建一个队列
Node* front = NULL;
Node* rear = NULL;
// 入队列中的元素
printf("Enqueuing 1, 2, 3 into the queue...\n");
enqueue(&front, &rear, 1);
enqueue(&front, &rear, 2);
enqueue(&front, &rear, 3);
// 出队列中的元素
printf("Dequeuing from the queue...\n");
dequeue(&front, &rear);
dequeue(&front, &rear);
dequeue(&front, &rear);
return 0;
}
```
阅读全文
相关推荐
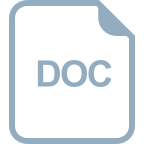
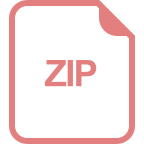
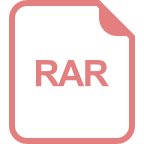
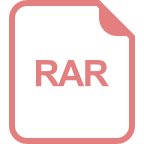
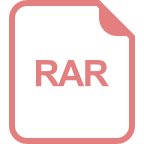
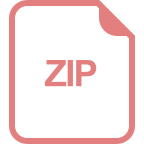
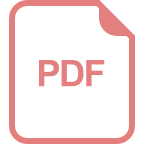
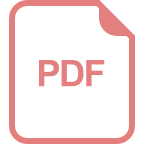
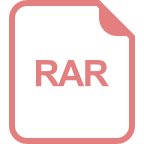
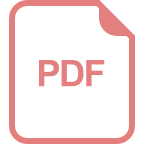
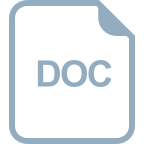
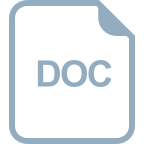
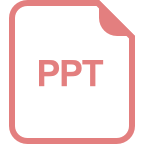
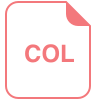
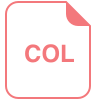