用c语言分模块详细写一遍
时间: 2024-03-10 09:49:41 浏览: 15
好的,下面我将用C语言分模块详细地写一遍学生成绩查询统计系统。
首先,我们需要定义三个结构体类型,包括`Student`、`Score`和`Grade`:
```c
// Student 结构体类型,表示学生信息
typedef struct {
char id[20]; // 学号
char name[20]; // 姓名
char gender[10]; // 性别
int age; // 年龄
char class[20]; // 班级
} Student;
// Score 结构体类型,表示成绩信息
typedef struct {
float chinese; // 语文成绩
float math; // 数学成绩
float english; // 英语成绩
float total; // 总分
float average; // 平均分
} Score;
// Grade 结构体类型,表示学生成绩信息
typedef struct {
Student student; // 学生信息
Score score; // 成绩信息
} Grade;
```
接着,我们将不同的功能模块分别封装到不同的函数中。
排序模块:为了实现根据学生姓名进行字典排序和根据成绩进行由高到低排序,我们可以采用快速排序算法。我们可以将排序算法封装到`sort`模块中,定义两个函数`sortByName`和`sortByScore`:
```c
// 对Grade类型的结构体数组按照学生姓名进行字典排序
void sortByName(Grade grades[], int left, int right) {
if (left >= right) {
return;
}
int i = left, j = right;
char* pivot = grades[(left + right) / 2].student.name;
while (i <= j) {
while (strcmp(grades[i].student.name, pivot) < 0) {
i++;
}
while (strcmp(grades[j].student.name, pivot) > 0) {
j--;
}
if (i <= j) {
Grade temp = grades[i];
grades[i] = grades[j];
grades[j] = temp;
i++;
j--;
}
}
sortByName(grades, left, j);
sortByName(grades, i, right);
}
// 对Grade类型的结构体数组按照成绩由高到低排序
void sortByScore(Grade grades[], int left, int right) {
if (left >= right) {
return;
}
int i = left, j = right;
float pivot = grades[(left + right) / 2].score.total;
while (i <= j) {
while (grades[i].score.total > pivot) {
i++;
}
while (grades[j].score.total < pivot) {
j--;
}
if (i <= j) {
Grade temp = grades[i];
grades[i] = grades[j];
grades[j] = temp;
i++;
j--;
}
}
sortByScore(grades, left, j);
sortByScore(grades, i, right);
}
```
查找模块:为了实现根据学号或姓名查找学生成绩,我们可以采用顺序查找算法。我们可以将查找算法封装到`search`模块中,定义一个函数`searchByKeyword`:
```c
// 在Grade类型的结构体数组中查找学号或姓名与关键字匹配的学生成绩信息
Grade* searchByKeyword(Grade grades[], int n, char* keyword) {
for (int i = 0; i < n; i++) {
if (strcmp(grades[i].student.id, keyword) == 0 || strcmp(grades[i].student.name, keyword) == 0) {
return &grades[i];
}
}
return NULL;
}
```
统计模块:为了实现统计各分数段(≥90分、80-89分、70-79分、60-69分、60分以下)的学生人数及其所占比例,并打印统计的结果,我们可以将统计算法封装到`stat`模块中,定义一个函数`statByScore`:
```c
// 统计Grade类型的结构体数组中各分数段的学生人数及其所占比例,并打印统计结果
void statByScore(Grade grades[], int n) {
int count[5] = {0}; // 各分数段学生人数的数组
for (int i = 0; i < n; i++) {
float score = grades[i].score.total;
if (score >= 90) {
count[0]++;
} else if (score >= 80) {
count[1]++;
} else if (score >= 70) {
count[2]++;
} else if (score >= 60) {
count[3]++;
} else {
count[4]++;
}
}
printf("≥90分: %d人,占比%.2f%%\n", count[0], (float)count[0] / n * 100);
printf("80-89分: %d人,占比%.2f%%\n", count[1], (float)count[1] / n * 100);
printf("70-79分: %d人,占比%.2f%%\n", count[2], (float)count[2] / n * 100);
printf("60-69分: %d人,占比%.2f%%\n", count[3], (float)count[3] / n * 100);
printf("60分以下: %d人,占比%.2f%%\n", count[4], (float)count[4] / n * 100);
}
```
最后,我们将所有的模块组合成一个完整的程序。我们可以将主函数放在`main`模块中,调用其他模块中的函数实现整个系统:
```c
// 主函数模块
#include <stdio.h>
#include <string.h>
#include "sort.h"
#include "search.h"
#include "stat.h"
#define MAX_STUDENT 100
int main() {
Grade grades[MAX_STUDENT];
int n = 0;
// 读取学生信息和成绩信息,存储到grades数组中
sortByName(grades, 0, n - 1);
sortByScore(grades, 0, n - 1);
char keyword[20];
printf("请输入要查找的学号或姓名:");
scanf("%s", keyword);
Grade* result = searchByKeyword(grades, n, keyword);
if (result) {
// 打印查找结果
} else {
printf("未找到相关信息\n");
}
statByScore(grades, n);
return 0;
}
```
完整的代码如下:
sort.h
```c
// 排序模块头文件
#ifndef SORT_H
#define SORT_H
#include "grade.h"
// 对Grade类型的结构体数组按照学生姓名进行字典排序
void sortByName(Grade grades[], int left, int right);
// 对Grade类型的结构体数组按照成绩由高到低排序
void sortByScore(Grade grades[], int left, int right);
#endif
```
sort.c
```c
// 排序模块实现文件
#include "sort.h"
#include <string.h>
// 对Grade类型的结构体数组按照学生姓名进行字典排序
void sortByName(Grade grades[], int left, int right) {
if (left >= right) {
return;
}
int i = left, j = right;
char* pivot = grades[(left + right) / 2].student.name;
while (i <= j) {
while (strcmp(grades[i].student.name, pivot) < 0) {
i++;
}
while (strcmp(grades[j].student.name, pivot) > 0) {
j--;
}
if (i <= j) {
Grade temp = grades[i];
grades[i] = grades[j];
grades[j] = temp;
i++;
j--;
}
}
sortByName(grades, left, j);
sortByName(grades, i, right);
}
// 对Grade类型的结构体数组按照成绩由高到低排序
void sortByScore(Grade grades[], int left, int right) {
if (left >= right) {
return;
}
int i = left, j = right;
float pivot = grades[(left + right) / 2].score.total;
while (i <= j) {
while (grades[i].score.total > pivot) {
i++;
}
while (grades[j].score.total < pivot) {
j--;
}
if (i <= j) {
Grade temp = grades[i];
grades[i] = grades[j];
grades[j] = temp;
i++;
j--;
}
}
sortByScore(grades, left, j);
sortByScore(grades, i, right);
}
```
search.h
```c
// 查找模块头文件
#ifndef SEARCH_H
#define SEARCH_H
#include "grade.h"
// 在Grade类型的结构体数组中查找学号或姓名与关键字匹配的学生成绩信息
Grade* searchByKeyword(Grade grades[], int n, char* keyword);
#endif
```
search.c
```c
// 查找模块实现文件
#include "search.h"
#include <string.h>
// 在Grade类型的结构体数组中查找学号或姓名与关键字匹配的学生成绩信息
Grade* searchByKeyword(Grade grades[], int n, char* keyword) {
for (int i =
相关推荐
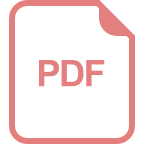
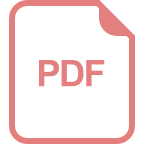















