php登陆注册页面mysql
时间: 2023-07-25 11:45:30 浏览: 53
好的,我可以为您提供一些基本的 PHP、MySQL 登录注册页面的代码示例,代码如下:
1. 注册页面(register.php)
```php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>注册页面</title>
</head>
<body>
<h2>用户注册</h2>
<form action="register.php" method="post">
<label>用户名</label>
<input type="text" name="username"><br><br>
<label>密码</label>
<input type="password" name="password"><br><br>
<label>确认密码</label>
<input type="password" name="confirm_password"><br><br>
<input type="submit" value="注册">
</form>
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// 获取用户输入的数据
$username = $_POST["username"];
$password = $_POST["password"];
$confirm_password = $_POST["confirm_password"];
// 验证用户输入的数据
if ($password != $confirm_password) {
echo "两次输入的密码不一致,请重新输入!";
} else {
// 连接数据库
$conn = mysqli_connect("localhost", "root", "root", "test");
// 判断连接是否成功
if (!$conn) {
die("连接失败:" . mysqli_connect_error());
}
// 将用户的数据插入到数据库中
$sql = "INSERT INTO users (username, password) VALUES ('$username', '$password')";
if (mysqli_query($conn, $sql)) {
echo "注册成功!";
} else {
echo "注册失败:" . mysqli_error($conn);
}
// 关闭数据库连接
mysqli_close($conn);
}
}
?>
</body>
</html>
```
2. 登录页面(login.php)
```php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>登录页面</title>
</head>
<body>
<h2>用户登录</h2>
<form action="login.php" method="post">
<label>用户名</label>
<input type="text" name="username"><br><br>
<label>密码</label>
<input type="password" name="password"><br><br>
<input type="submit" value="登录">
</form>
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// 获取用户输入的数据
$username = $_POST["username"];
$password = $_POST["password"];
// 连接数据库
$conn = mysqli_connect("localhost", "root", "root", "test");
// 判断连接是否成功
if (!$conn) {
die("连接失败:" . mysqli_connect_error());
}
// 查询数据库中是否存在该用户
$sql = "SELECT * FROM users WHERE username='$username' AND password='$password'";
$result = mysqli_query($conn, $sql);
if (mysqli_num_rows($result) == 1) {
// 登录成功,跳转到首页
header("Location: index.php");
} else {
// 登录失败,提示错误信息
echo "登录失败:用户名或密码错误!";
}
// 关闭数据库连接
mysqli_close($conn);
}
?>
</body>
</html>
```
注:以上代码仅供参考,具体的实现方式和细节可能会因为不同的需求而不同。
相关推荐
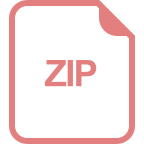
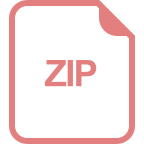














