spring.cloud.gateway.routes.predicates.args
时间: 2024-04-09 08:20:58 浏览: 160
`spring.cloud.gateway.routes.predicates.args` 是 Spring Cloud Gateway 的一个属性,用于配置路由的谓词参数。在 Gateway 中,路由的谓词用于匹配请求,如果请求匹配成功,就会将请求转发到相应的目标服务。
`spring.cloud.gateway.routes.predicates.args` 属性允许你为谓词传递一些参数,以便更精确地匹配请求。例如,如果你使用了 `Path` 谓词来匹配请求路径,可以使用 `spring.cloud.gateway.routes.predicates.args.pattern` 参数来指定匹配模式。
举个例子,下面的配置使用 `Path` 谓词来匹配请求路径,只有当请求路径以 `/api` 开头时才会进行路由:
```yaml
spring:
cloud:
gateway:
routes:
- id: myroute
uri: https://example.com
predicates:
- Path=/api/**
args:
pattern: "/api/**"
```
在上面的配置中,`Path=/api/**` 表示使用 `Path` 谓词匹配请求路径,`args` 属性中的 `pattern: "/api/**"` 则指定了匹配模式。
相关问题
springcloud gateway 怎么从redis动态获取 gateway routes
在Spring Cloud Gateway中,你可以使用Redis来实现动态获取Gateway的路由信息。下面是一个基本的实现思路:
1. **配置Redis**:首先,确保你已经正确配置了Redis,并能够通过Spring Boot进行连接和操作。你可以参考Spring Data Redis的官方文档来了解如何配置和使用Redis。
2. **创建自定义的RouteDefinitionLocator**:创建一个自定义的`RouteDefinitionLocator`实现,用于从Redis中获取路由信息。这个实现类需要实现`getRouteDefinitions()`方法,并在该方法中从Redis中获取路由配置信息。
```java
@Component
public class RedisRouteDefinitionLocator implements RouteDefinitionLocator {
private final RedisTemplate<String, Object> redisTemplate;
public RedisRouteDefinitionLocator(RedisTemplate<String, Object> redisTemplate) {
this.redisTemplate = redisTemplate;
}
@Override
public Flux<RouteDefinition> getRouteDefinitions() {
List<RouteDefinition> routeDefinitions = new ArrayList<>();
// 从Redis中获取路由配置信息,并将其转换为RouteDefinition对象
// 将解析后的RouteDefinition对象添加到routeDefinitions列表中
return Flux.fromIterable(routeDefinitions);
}
}
```
3. **配置Gateway使用自定义的RouteDefinitionLocator**:在配置类中,将自定义的`RouteDefinitionLocator`注册为Bean,并将其设置为Gateway的`RouteDefinitionLocator`。
```java
@Configuration
public class GatewayConfig {
@Bean
public RedisTemplate<String, Object> redisTemplate() {
// 创建和配置RedisTemplate实例
return redisTemplate;
}
@Bean
public RouteDefinitionLocator routeDefinitionLocator(RedisTemplate<String, Object> redisTemplate) {
return new RedisRouteDefinitionLocator(redisTemplate);
}
@Bean
public RouteDefinitionLocatorComposite routeDefinitionLocatorComposite(RouteDefinitionLocator... locators) {
return new RouteDefinitionLocatorComposite(Arrays.asList(locators));
}
}
```
在上述配置中,`RedisTemplate`是一个用于与Redis进行交互的Spring Data Redis的组件。你可以根据实际情况进行配置和使用。
4. **配置Redis中的路由信息**:在Redis中存储路由信息,可以使用Hash结构来存储每个路由的详细信息,例如路由ID、URI、谓词、过滤器等。
```shell
HSET gateway_routes route1 '{ "id": "route1", "uri": "http://example.com", "predicates": [{ "name": "Path", "args": { "pattern": "/example" } }] }'
HSET gateway_routes route2 '{ "id": "route2", "uri": "http://example.org", "predicates": [{ "name": "Path", "args": { "pattern": "/example2" } }] }'
```
上述示例中,使用Hash结构存储了两个路由的详细信息,每个路由信息以JSON格式表示。
通过以上步骤,你就可以通过Redis动态获取Gateway的路由信息了。每当路由配置信息在Redis中发生变化时,Gateway会自动重新加载路由信息。
需要注意的是,上述示例仅提供了一个基本的思路和示例代码,你可能需要根据实际需求进行适当的调整和扩展。
希望以上内容对你有所帮助!
Spring Cloud Gateway 整合Spring Security
Spring Cloud Gateway 和 Spring Security 可以相互整合,以提供安全的 API 网关解决方案。下面是一些步骤来实现这个整合:
1. 首先,在你的 Spring Cloud Gateway 项目中添加 Spring Security 的依赖。你可以在 `build.gradle` 或 `pom.xml` 文件中添加以下依赖:
```groovy
implementation 'org.springframework.boot:spring-boot-starter-security'
```
2. 创建一个安全配置类来配置 Spring Security。你可以创建一个类,并使用 `@EnableWebSecurity` 注解标记它,然后扩展 `WebSecurityConfigurerAdapter` 类。在这个类中,你可以配置认证和授权规则。
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/api/public/**").permitAll() // 允许公共访问的接口
.anyRequest().authenticated() // 其他接口需要认证
.and()
.httpBasic(); // 使用 HTTP Basic 认证
}
}
```
3. 在 Gateway 中配置 Spring Security。在你的 Gateway 配置文件(如 `application.yml`)中,添加以下配置:
```yaml
spring:
security:
user:
name: user # 设置默认用户名
password: password # 设置默认密码
```
4. 进一步配置 Gateway 的路由和鉴权规则。你可以使用 Gateway 的路由配置来定义每个路由的鉴权规则。例如,你可以为需要认证的路由添加 `filters`,进行基于角色的访问控制:
```yaml
spring:
cloud:
gateway:
routes:
- id: my_route
uri: http://example.com
predicates:
- Path=/api/private/**
filters:
- name: Security
args:
roles: ROLE_ADMIN
```
在上面的例子中,只有具有 `ROLE_ADMIN` 角色的用户才能访问 `/api/private/**` 路径。
以上是整合 Spring Cloud Gateway 和 Spring Security 的基本步骤。你可以根据自己的需求进一步定制和配置。
阅读全文
相关推荐
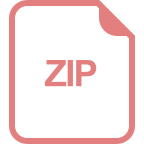
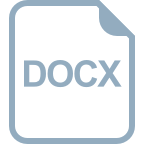
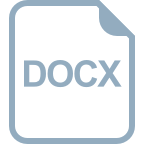
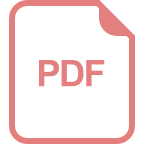
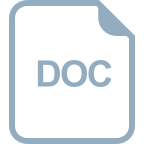
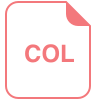
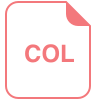
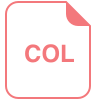
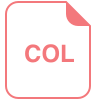
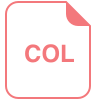






