编写Linux shell脚本使得依次执行./my_cd ./my_dir -l ./my_md test11 ./my_md test22 ./my_md test33 ./my_del test1.txt ./my_copy test2.txt test222.txt ./my_xcopy test3.txt test333.txt ./my_move test222.txt test22 ./my_rd test11 ./my_ren test333.txt test123.txt ./my_type test123.txt
时间: 2023-07-15 14:11:51 浏览: 217
可以尝试编写以下脚本:
```
#!/bin/bash
# 执行 my_cd
./my_cd
# 执行 my_dir -l
./my_dir -l
# 执行 my_md test11
./my_md test11
# 执行 my_md test22
./my_md test22
# 执行 my_md test33
./my_md test33
# 执行 my_del test1.txt
./my_del test1.txt
# 执行 my_copy test2.txt test222.txt
./my_copy test2.txt test222.txt
# 执行 my_xcopy test3.txt test333.txt
./my_xcopy test3.txt test333.txt
# 执行 my_move test222.txt test22
./my_move test222.txt test22
# 执行 my_rd test11
./my_rd test11
# 执行 my_ren test333.txt test123.txt
./my_ren test333.txt test123.txt
# 执行 my_type test123.txt
./my_type test123.txt
```
注意,以上脚本只是一个简单的示例,假设执行这些命令不会出现任何错误。如果要考虑错误处理,可以在每个命令后添加一些逻辑来检查命令是否成功执行,并相应地处理错误情况。
相关问题
写一个shell脚本,遍历当前目录下除了models testbench所有的文件夹,文件夹下只保留test.sim_command test.startsim vtb_scenario.f,其余文件全部删除
当你需要编写一个Shell脚本来遍历当前目录下的所有文件夹,并且仅保留特定的几个文件而删除其他文件时,可以使用以下bash script作为基础模板:
```bash
#!/bin/bash
# 遍历当前目录及其子目录
for dir in $(find . -type d); do
# 判断是否为models或testbench文件夹
if [[ $dir != "models" && $dir != "testbench" ]]; then
echo "Cleaning directory: $dir"
# 进入该文件夹并查找需要保留的文件
cd "$dir"
files_to_keep=("test.sim_command" "test.startsim" "vtb_scenario.f")
for file in *; do
# 如果文件不在保留列表中,则删除
if ! [[ "${files_to_keep[*]}" =~ "$file" ]]; then
rm "$file"
fi
done
# 返回到上一级目录
cd ..
fi
done
echo "Cleanup completed."
写一个shell脚本,遍历当前目录下,除了models 或者testbench外所有的文件夹,文件夹下只保留test.sim_command test.startsim vtb_scenario.f,其余文件全部删除
你可以创建一个名为`remove_extra_files.sh`的shell脚本来完成这个任务。下面是一个示例脚本,它会遍历当前目录及其子目录,排除名为"models"和"testbench"的文件夹,并保留特定的三个文件:
```bash
#!/bin/bash
# 遍历当前目录下的所有文件夹,但跳过指定的两个
for dir in */!(models|testbench) ; do
# 访问每个文件夹
cd "$dir"
# 检查并保留特定文件
if [ -e "test.sim_command" ] && [ -e "test.startsim" ] && [ -e "vtb_scenario.f" ]; then
echo "Keeping files in directory $dir"
else
# 删除除指定文件外的所有内容
find . -maxdepth 1 -type f ! -name 'test.sim_command' ! -name 'test.startsim' ! -name 'vtb_scenario.f' | xargs rm -f
echo "Deleting extra files from directory $dir"
fi
# 返回上一级目录
cd ..
done
echo "Script finished."
```
要在终端运行此脚本,你需要先保存它,然后赋予执行权限:
```sh
chmod +x remove_extra_files.sh
./remove_extra_files.sh
```
阅读全文
相关推荐
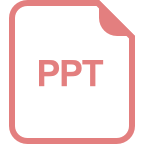
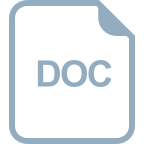
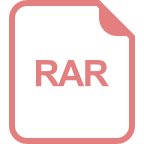
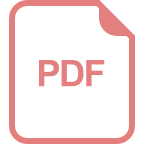
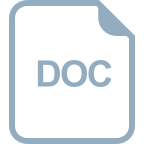
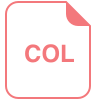
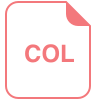
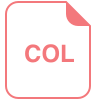
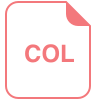





