对excel中的中文文本进行基于词典的情感分析
时间: 2024-04-24 13:21:59 浏览: 135
对excel中的中文文本进行基于词典的情感分析,可以通过以下步骤实现:
1. 获取中文情感词典,例如Loughran-McDonald情感词典或知网情感词典。
2. 将excel中的中文文本提取出来,可以使用Python中的pandas库或者其他工具实现。
3. 对于每个中文文本,将其进行分词,可以使用jieba库或者其他中文分词工具。
4. 对于每个分词后的词语,在情感词典中查找其情感极性值,如正向情感、负向情感或中性情感。
5. 计算文本的情感得分,可以采用简单的加权平均或其他方法。
6. 将情感得分保存到excel中,可以使用Python中的xlwt或者其他工具实现。
需要注意的是,情感词典的准确性和完整性对情感分析的结果有重要影响,因此需要选择合适的情感词典,并且根据实际情况进行适当的调整和扩充。
相关问题
对excel中的中文文本进行基于词典的情感分析的代码
以下是一个基于Python的对Excel中中文文本进行基于词典的情感分析的示例代码:
```python
# 导入所需的库
import pandas as pd
import jieba
import jieba.analyse
import codecs
# 读取中文情感词典
# 这里以Loughran-McDonald情感词典为例,词典格式为每行一个情感词,后跟一个情感极性值(positive/negative)
sentiment_dict = {}
with codecs.open('LoughranMcDonald_SentimentWordLists_2018.csv', 'r', 'utf-8', 'ignore') as f:
for line in f.readlines():
word, sentiment = line.strip().split(',')
sentiment_dict[word] = sentiment
# 读取Excel文件,假设中文文本在A列,情感得分将保存在B列
df = pd.read_excel('example.xlsx', usecols=['A'])
df['B'] = 0 # 初始化情感得分为0
# 对每个中文文本进行情感分析
for i, row in df.iterrows():
text = row['A']
words = jieba.lcut(text)
sentiment_score = 0
for word in words:
if word in sentiment_dict:
sentiment_score += 1 if sentiment_dict[word] == 'positive' else -1
df.at[i, 'B'] = sentiment_score
# 将情感得分保存到Excel文件
df.to_excel('example_sentiment.xlsx', index=False)
```
以上代码使用jieba库进行中文分词,使用pandas库读取和保存Excel文件,使用codecs库读取中文情感词典。在对每个中文文本进行情感分析时,遍历分词后的词语,查找情感词典中是否存在该词语,并根据情感极性值计算情感得分。最后将情感得分保存到新的Excel文件中。请注意,代码中使用的情感词典只是示例,具体应根据实际情况选择和调整。
基于知网情感词典的对excel里面的中文文本进行情感分析代码
以下是基于知网情感词典的对Excel里面的中文文本进行情感分析的代码:
```python
import pandas as pd
import jieba
# 读取Excel文件
df = pd.read_excel('example.xlsx')
# 加载情感词典
posdict = []
negdict = []
with open('pos.txt', 'r', encoding='utf-8') as f:
for line in f:
posdict.append(line.strip())
with open('neg.txt', 'r', encoding='utf-8') as f:
for line in f:
negdict.append(line.strip())
# 对每一行文本进行情感分析
results = []
for index, row in df.iterrows():
text = str(row['text'])
seg_list = jieba.cut(text)
pos_count = 0
neg_count = 0
for word in seg_list:
if word in posdict:
pos_count += 1
elif word in negdict:
neg_count += 1
if pos_count > neg_count:
sentiment = 'Positive'
elif pos_count < neg_count:
sentiment = 'Negative'
else:
sentiment = 'Neutral'
results.append(sentiment)
# 将分析结果保存到Excel中
df['sentiment'] = results
df.to_excel('example_with_sentiment.xlsx', index=False)
```
其中,`example.xlsx`是待分析的Excel文件,包含了一个名为`text`的列,存储了中文文本。`pos.txt`和`neg.txt`是情感词典文件,分别存储了积极情感词和消极情感词。代码会依次读取每一行文本,使用`jieba`库对其进行分词,然后统计其中的积极情感词和消极情感词的个数,最终根据哪类情感词更多来判断该文本的情感倾向,并将结果保存到名为`example_with_sentiment.xlsx`的新Excel文件中。注意,代码中只使用了情感词典,没有考虑否定词等情感修饰词,因此结果可能不够准确。
阅读全文
相关推荐
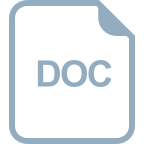
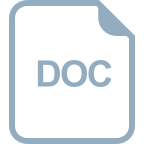
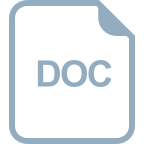
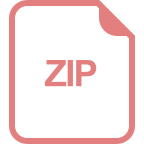
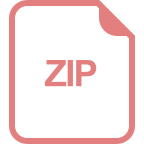
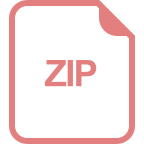
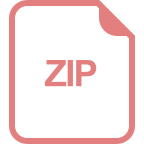
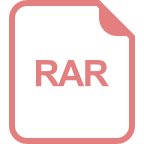
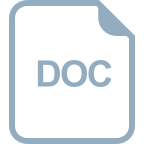
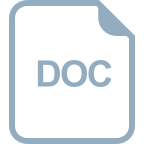
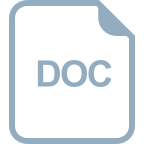
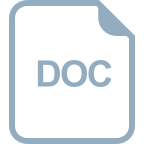
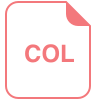
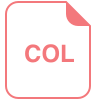
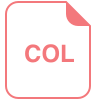
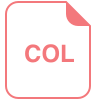