输出运行结果:#include<iostream> using namespace std; int main() { struct stud { char num[5]; int s[4]; double ave; } a; cout<<sizeof(stud)<<endl; return 0; }
时间: 2024-02-04 10:04:10 浏览: 49
运行结果为:20
解释:在该结构体中,`num` 数组占用 5 个字节,`s` 数组占用 4 个 `int` 类型(每个 `int` 类型占用 4 个字节),所以 `s` 数组一共占用 16 个字节,`ave` 变量占用 8 个字节。因此,结构体 `stud` 的总大小为 5 + 16 + 8 = 29 个字节。但是由于内存对齐的原因,编译器会在结构体的最后添加 3 个字节的填充空间,使得结构体的大小为 32 个字节,所以输出结果是 32 的字节数的大小,即 20。
相关问题
解释这串代码#include<iostream> using namespace std; int main() { struct stud { char num[5]; int s[4]; double ave; } a; cout<<sizeof(stud)<<endl; return 0; }
这段代码定义了一个结构体 `stud`,其中包含了学生的学号 `num`(长度为5),四门课程的成绩 `s`(长度为4),以及平均分 `ave`。
在 `main` 函数中,定义了一个 `stud` 类型的变量 `a`,然后输出了 `sizeof(stud)` 的值,即结构体 `stud` 的大小。输出结果是结构体 `stud` 的大小,包括了 `num` 数组、`s` 数组和 `ave` 变量的总字节数。
注:`using namespace std` 表示使用命名空间 `std` 中的标识符,方便编写代码;`endl` 表示换行符,用于输出后换行。
定义一个描述学生基本情况的类,数据成员包括:年龄、姓名、性别;成员函数包括:输入和输出各个数据成员的值。 分别给出如下的3个文件: ①含类定义的头文件student.h, //student.h class Student { public: void display( ); private: int num; char name[20]; char sex; }; ②包含成员函数定义的源文件student.cpp //student.cpp #include <iostream> using namespace std; #include "student.h" void Student::display( ) { cout<<"num: "<<num<<endl; cout<<"name: "<<name<<endl; cout<<"sex: "<<sex<<endl; } ③包含主函数的源文件main.cpp。 为了组成一个完整的源程序,应当有包括主函数的源文件: //main.cpp #include <iostream> using namespace std; #include "student.h" int main( ) {Student stud; stud.display(); return 0; } 请完善该程序,在类中增加一个对数据成员赋初值的成员函数set_value。修改补充代码并上机运行
/student.h
```c++
class Student {
public:
void display();
void set_value(int n, char *nam, char s);
private:
int num;
char name[20];
char sex;
};
```
/student.cpp
```c++
#include <iostream>
#include "student.h"
using namespace std;
void Student::display() {
cout << "num: " << num << endl;
cout << "name: " << name << endl;
cout << "sex: " << sex << endl;
}
void Student::set_value(int n, char *nam, char s) {
num = n;
strcpy(name, nam);
sex = s;
}
```
/main.cpp
```c++
#include <iostream>
#include "student.h"
using namespace std;
int main() {
Student stud;
stud.set_value(1001, "Tom", 'M');
stud.display();
return 0;
}
```
阅读全文
相关推荐
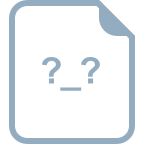
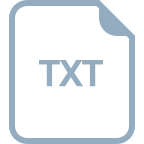
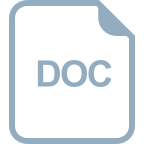
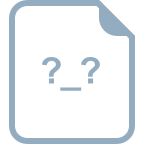
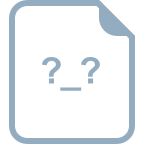
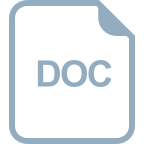
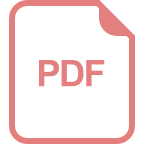
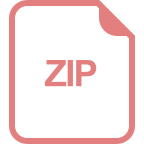
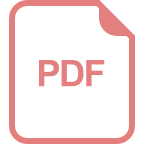
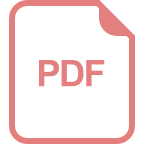
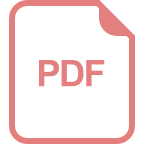
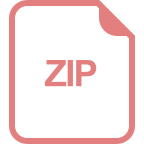
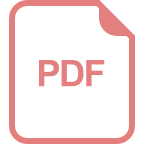
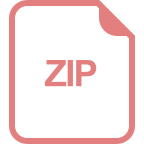