利用javascript实现:输入一个整数n,再输入n个整数,按照输入的顺序建立单链表,并遍历所建立的单链表,输出这些数据。 输入格式: 测试数据有多组,处理到文件尾。每组测试输入一个整数n,再输入n个整数。 输出格式: 对于每组测试,输出顺序链表中的各结点的数据域的值(数据之间留一个空格)。 输入样例: 5 1 2 3 4 5 输出样例: 5 4 3 2 1
时间: 2023-02-17 17:46:45 浏览: 55
以下是使用 JavaScript 实现题目要求的代码示例:
```
const readline = require('readline');
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
// 定义单链表节点
class Node {
constructor(val) {
this.val = val;
this.next = null;
}
}
// 定义单链表
class LinkedList {
constructor() {
this.head = null;
this.size = 0;
}
// 向单链表中添加元素
add(val) {
const newNode = new Node(val);
if (this.head === null) {
this.head = newNode;
} else {
let current = this.head;
while (current.next !== null) {
current = current.next;
}
current.next = newNode;
}
this.size++;
}
// 遍历单链表
traverse() {
let current = this.head;
while (current !== null) {
console.log(current.val);
current = current.next;
}
}
}
// 读取用户输入,建立单链表并遍历
rl.on('line', (line) => {
const list = new LinkedList();
const nums = line.split(' ').map(num => parseInt(num, 10));
const n = nums[0];
for (let i = 1; i <= n; i++) {
list.add(nums[i]);
}
list.traverse();
});
```
上述代码定义了 `Node` 类和 `LinkedList` 类,前者表示单链表中的节点,后者表示单链表。我们可以使用 `readline` 模块来读取用户输入,然后用读入的数据来建立单链表,最后遍历该单链表输出每个节点的数据域的值。
相关推荐
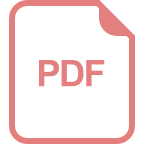
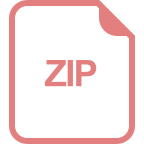














