java实现批量读取excel文档并替换文档中的指定字符串
时间: 2024-02-17 09:09:20 浏览: 28
你可以使用Apache POI库来实现批量读取Excel文档并替换文档中的指定字符串。
以下是一个示例代码,假设你要替换的字符串是"oldString",替换为"newString":
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelReplace {
public static void main(String[] args) throws IOException {
String folderPath = "/path/to/folder"; // 存放Excel文件的文件夹路径
String oldString = "oldString"; // 需要替换的字符串
String newString = "newString"; // 替换后的字符串
File folder = new File(folderPath);
File[] files = folder.listFiles();
for (File file : files) {
if (file.isFile() && file.getName().endsWith(".xlsx")) {
FileInputStream inputStream = new FileInputStream(file);
Workbook workbook = new XSSFWorkbook(inputStream);
Sheet sheet = workbook.getSheetAt(0);
for (Row row : sheet) {
for (Cell cell : row) {
if (cell.getCellType() == Cell.CELL_TYPE_STRING) {
String cellValue = cell.getStringCellValue();
if (cellValue.contains(oldString)) {
cell.setCellValue(cellValue.replace(oldString, newString));
}
}
}
}
inputStream.close();
FileOutputStream outputStream = new FileOutputStream(file);
workbook.write(outputStream);
outputStream.close();
workbook.close();
}
}
}
}
```
这个代码会遍历指定文件夹下的所有Excel文件,读取第一个Sheet中的所有单元格,如果单元格中包含需要替换的字符串,就将其替换为新的字符串。最后,将修改后的文档保存回原来的文件中。
相关推荐
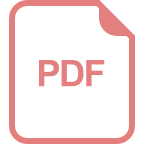
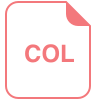
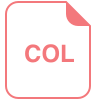
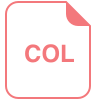
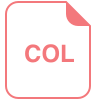
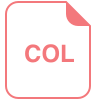









