python rsa算法
时间: 2024-01-11 22:22:45 浏览: 89
RSA算法是一种非对称加密算法,它使用一对公钥和私钥来进行加密和解密。下面是一个基于Python实现RSA算法的例子:
```python
import random
# 判断一个数是否为素数
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
# 判断两个数是否互为素数
def is_coprime(a, b):
return gcd(a, b) == 1
# 欧几里得算法求最大公约数
def gcd(a, b):
while b != 0:
a, b = b, a % b
return a
# 扩展欧几里得算法求模逆
def mod_inverse(a, m):
if gcd(a, m) != 1:
return None
u1, u2, u3 = 1, 0, a
v1, v2, v3 = 0, 1, m
while v3 != 0:
q = u3 // v3
v1, v2, v3, u1, u2, u3 = (u1 - q * v1), (u2 - q * v2), (u3 - q * v3), v1, v2, v3
return u1 % m
# 产生公私钥
def generate_keys(p, q):
if not (is_prime(p) and is_prime(q)):
return None
n = p * q
phi = (p - 1) * (q - 1)
e = random.randrange(1, phi)
while not is_coprime(e, phi):
e = random.randrange(1, phi)
d = mod_inverse(e, phi)
return ((e, n), (d, n))
# 加密明文
def encrypt(plaintext, public_key):
e, n = public_key
ciphertext = [pow(ord(char), e, n) for char in plaintext]
return ciphertext
# 解密密文
def decrypt(ciphertext, private_key):
d, n = private_key
plaintext = [chr(pow(char, d, n)) for char in ciphertext]
return ''.join(plaintext)
# 代码测试
p = 61
q = 53
keys = generate_keys(p, q)
public_key, private_key = keys
plaintext = "Hello, RSA!"
ciphertext = encrypt(plaintext, public_key)
decrypted_text = decrypt(ciphertext, private_key)
print("Public key:", public_key)
print("Private key:", private_key)
print("Ciphertext:", ciphertext)
print("Decrypted text:", decrypted_text)
```
这段代码实现了RSA算法的各个步骤,包括判断素数、判断互为素数、欧几里得算法求最大公约数、扩展欧几里得算法求模逆、产生公私钥、加密明文和解密密文。你可以根据需要修改其中的参数和明文来进行测试。
阅读全文
相关推荐
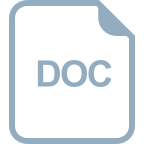
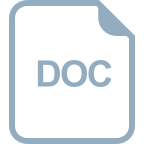
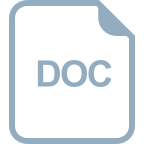
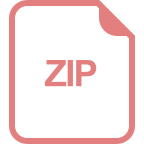
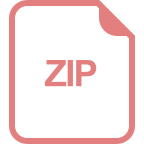
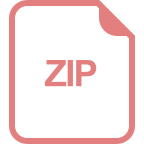
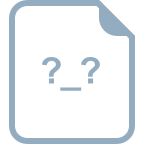
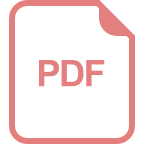









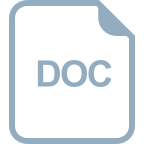
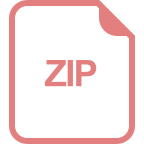