用python写一个钟表,可以随着时间指针转动
时间: 2024-05-08 21:16:01 浏览: 15
这是一个比较复杂的项目,需要使用Python的图形化库,比如Tkinter或Pygame。以下是使用Tkinter编写的一个钟表示例代码:
```python
from tkinter import *
import time
class Clock:
def __init__(self, root):
self.root = root
self.root.title("Clock")
self.canvas = Canvas(root, width=300, height=300)
self.canvas.pack()
self.draw_clock()
def draw_clock(self):
self.canvas.delete("all")
# Draw clock face
self.canvas.create_oval(50, 50, 250, 250, width=2)
# Draw hour markers
for i in range(12):
x1 = 150 + 100 * math.cos(math.radians(i * 30 - 90))
y1 = 150 + 100 * math.sin(math.radians(i * 30 - 90))
x2 = 150 + 90 * math.cos(math.radians(i * 30 - 90))
y2 = 150 + 90 * math.sin(math.radians(i * 30 - 90))
self.canvas.create_line(x1, y1, x2, y2, width=2)
# Draw minute markers
for i in range(60):
x1 = 150 + 100 * math.cos(math.radians(i * 6 - 90))
y1 = 150 + 100 * math.sin(math.radians(i * 6 - 90))
x2 = 150 + 95 * math.cos(math.radians(i * 6 - 90))
y2 = 150 + 95 * math.sin(math.radians(i * 6 - 90))
self.canvas.create_line(x1, y1, x2, y2, width=1)
# Draw hour hand
hour = int(time.strftime("%I"))
minute = int(time.strftime("%M"))
hour_angle = (hour + minute / 60) * 30 - 90
x = 150 + 50 * math.cos(math.radians(hour_angle))
y = 150 + 50 * math.sin(math.radians(hour_angle))
self.canvas.create_line(150, 150, x, y, width=4)
# Draw minute hand
minute_angle = minute * 6 - 90
x = 150 + 80 * math.cos(math.radians(minute_angle))
y = 150 + 80 * math.sin(math.radians(minute_angle))
self.canvas.create_line(150, 150, x, y, width=2)
# Draw second hand
second_angle = int(time.strftime("%S")) * 6 - 90
x = 150 + 90 * math.cos(math.radians(second_angle))
y = 150 + 90 * math.sin(math.radians(second_angle))
self.canvas.create_line(150, 150, x, y, width=1)
# Update every second
self.root.after(1000, self.draw_clock)
root = Tk()
clock = Clock(root)
root.mainloop()
```
在这个示例中,我们使用了Tkinter的Canvas小部件来绘制钟表的图形。我们首先绘制了钟表的外形,然后绘制了小时和分钟的标记。接下来,我们使用当前的时间计算出时针、分针和秒针的角度,并绘制它们的线条。最后,我们使用`after`方法每秒更新一次钟表。
请注意,这个示例只是一个基本的钟表,你可以根据自己的需要进行调整和扩展。
相关推荐
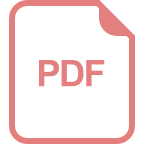
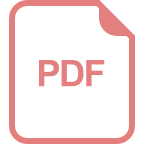














