import cv2 from matplotlib import pyplot as plt import numpy as np image = cv2.imread("0.jpg") gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) ret, binary = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY) contours, hierarchy = cv2.findContours(binary, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE) cv2.drawContours(image, contours, -1, (255, 0, 0), 2) cnt_len = cv2.arcLength(contours[0], True) cnt = cv2.approxPolyDP(contours[0], 0.02*cnt_len, True) if len(cnt) == 4: cv2.drawContours(image, [cnt], -1, (255, 255, 0), 3 ) plt.imshow(image) plt.show()
时间: 2023-08-13 08:34:11 浏览: 40
这段代码使用 OpenCV 和 Matplotlib 库读取图像,进行二值化和轮廓查找,并用蓝色绘制所有的轮廓,最后用黄色绘制符合条件的四边形轮廓。你需要注意的是,这段代码仅适用于图像中只有一个四边形的情况,如果有多个四边形或不止一个轮廓,代码需要进行相应的修改。
以下是代码中各个函数的作用:
- cv2.imread:读取图像
- cv2.cvtColor:将图像转换为灰度图像
- cv2.threshold:二值化图像
- cv2.findContours:查找轮廓
- cv2.drawContours:绘制轮廓
- cv2.arcLength:计算轮廓周长
- cv2.approxPolyDP:拟合多边形
以下是代码:
```python
import cv2
from matplotlib import pyplot as plt
import numpy as np
# 读取图像
image = cv2.imread("0.jpg")
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 二值化
ret, binary = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
# 查找轮廓
contours, hierarchy = cv2.findContours(binary, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 绘制所有轮廓
cv2.drawContours(image, contours, -1, (255, 0, 0), 2)
# 拟合多边形
cnt_len = cv2.arcLength(contours[0], True)
cnt = cv2.approxPolyDP(contours[0], 0.02*cnt_len, True)
# 绘制符合条件的四边形轮廓
if len(cnt) == 4:
cv2.drawContours(image, [cnt], -1, (255, 255, 0), 3 )
# 显示图像
plt.imshow(image)
plt.show()
```
代码中使用了很多 OpenCV 的函数,如果对这些函数不熟悉,可以先去学习一下基础知识。
相关推荐
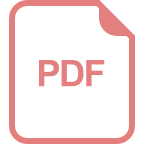
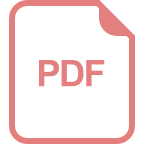
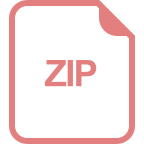















