QT图像处理对比度的代码
时间: 2024-08-11 07:01:17 浏览: 67
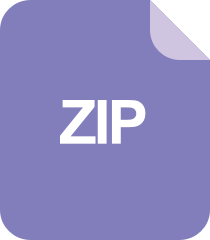
基于QT5+Opencv完成简单的图像处理及视频处理软件
QT图像处理库中,我们可以利用`QImage`和`QColor`等类来进行图像操作,包括改变图像的对比度。下面是一个简单的例子说明如何通过调整像素值的方式改变图像的对比度:
```cpp
#include <QImage>
#include <QDebug>
// 用于计算新像素值的函数
int adjustContrast(int pixelValue) {
// 假设原始最大值是255(对于8位图像)
int maxVal = 255;
// 可以调整此值以改变对比度(0到1之间的值会降低对比度,大于1会增强对比度)
float contrastFactor = 1.6f;
return qBound(0, qMax(0, qMin(maxVal, static_cast<int>(pixelValue * contrastFactor))), maxVal);
}
void changeContrast(const QImage &sourceImage, QImage &destinationImage, float contrastFactor) {
if (sourceImage.format() != QImage::Format_RGB888) {
qDebug() << "Source image format is not RGB888";
return;
}
destinationImage = sourceImage.copy();
for (int y = 0; y < destinationImage.height(); ++y) {
for (int x = 0; x < destinationImage.width(); ++x) {
QRgb color = destinationImage.pixel(x, y);
int newRed = adjustContrast(qRed(color));
int newGreen = adjustContrast(qGreen(color));
int newBlue = adjustContrast(qBlue(color));
QColor newColor(newRed, newGreen, newBlue);
destinationImage.setPixel(x, y, newColor.rgb());
}
}
}
```
### 使用示例
你可以将上述`changeContrast`函数集成到你的项目中,并传递你需要调整对比度的源图像和目标图像,以及所需的对比度系数(通常是在0.8到1.2之间),来达到所需的效果。
### 相关问题:
1. 是否有其他方法可以调整图像的对比度而不仅仅是通过修改像素值?
2. 调整图像对比度的最佳实践是什么?
3. 如果我的图像颜色空间不是RGB,如何适配这个代码?
阅读全文
相关推荐
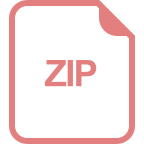
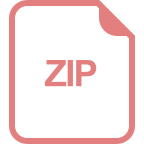
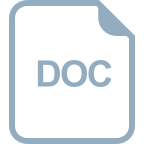
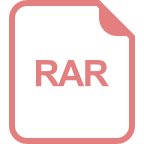
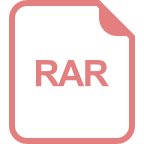
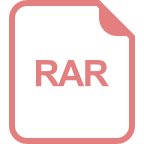
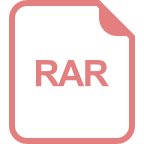
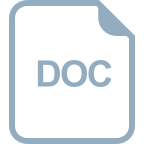
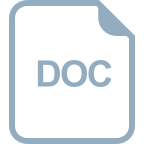
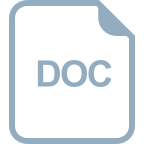
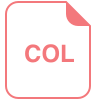
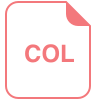
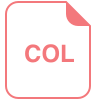
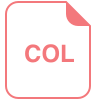
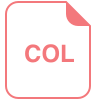
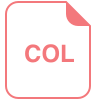
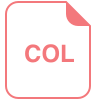
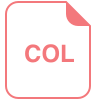