c语言实现encodeURIComponent
时间: 2024-08-08 16:01:16 浏览: 51
`encodeURIComponent()`是一个JavaScript内置函数,用于对URL组件进行编码,以确保它们能在URL中安全地传输。这个函数接受一个字符串作为参数,并返回一个已编码的版本。它将特殊字符替换为%加上该字符的十六进制ASCII码表示。
在C语言中,虽然没有直接对应的`encodeURIComponent()`函数,但我们可以通过手动实现编码过程来达到类似的效果。以下是使用标准库函数完成此任务的一种方法:
```c
#include <stdio.h>
#include <string.h>
void encodeURIComponent(const char *input, char *output) {
const char *in = input;
int i = 0; // index for output string
while (*in) {
switch (*in) {
case ' ': // Space character
*(output + i++) = '+';
break;
default:
if (isalnum(*in)) {
*(output + i++) = *in;
} else {
// Hexadecimal representation of the ASCII code
sprintf(output + i, "%2X", *in);
i += 2;
}
break;
}
++in;
}
*(output + i) = '\0'; // Null terminate the encoded string
}
int main() {
const char *input = "Hello World! How are you?";
char output[50] = "";
encodeURIComponent(input, output);
printf("Encoded URL Component:\n%s\n", output);
return 0;
}
```
这段代码首先导入了必要的头文件,然后定义了一个名为`encodeURIComponent`的函数,它接收原始输入字符串以及编码后的结果存储地址。在内部循环遍历输入字符串的每个字符。如果字符是空格,则将其替换为加号(`+`);对于字母数字字符,直接复制到输出缓冲区;对于其他字符(包括一些特殊符号),使用`sprintf`函数将其转换为其十六进制ASCII码表示形式。
在这个例子中,`main`函数提供了一个测试用的字符串,调用`encodeURIComponent`对其进行处理,并打印出编码后的结果。
---
阅读全文
相关推荐
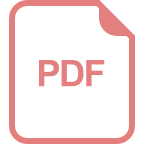
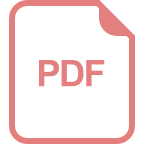
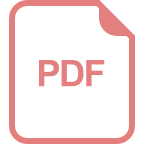

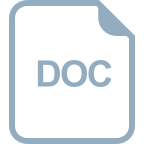











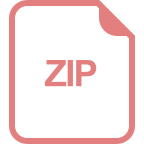
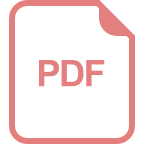