C语言标准函数库中包括 strstr 函数,在主串中查找子串。作为练习,我们自己编写一个功能与之相同的函数。 函数原型 char* StrStr(const char *txt, const char *pat); 说明:txt 和 pat 分别为主串和子串的起始地址。若查找成功,则函数值为子串在主串中首次出现的起始地址,否则函数值为NULL。 特别地,我们对C语言库函数strstr进行适当修改:若子串为空串,则没有意义,函数值规定为NULL。 裁判程序 #include <stdio.h> #include <string.h> char* StrStr(const char *txt, const char *pat); int main() { char m[1024], s[1024], *p; gets(m); gets(s); p = StrStr(m, s); if (p) { printf("%d\n", p - m); } else { puts("NULL"); } return 0; } /* 你提交的代码将被嵌在这里 */ 输入样例1 This is a pencil is 输出样例1 2 输入样例2 This is a pencil be 输出样例2 NULL
时间: 2024-03-10 20:50:08 浏览: 118
以下是一个实现功能与 `strstr` 函数相同的 `StrStr` 函数的示例代码:
```c
char* StrStr(const char *txt, const char *pat) {
if (*pat == '\0') { // 若子串为空串,则返回 NULL
return NULL;
}
while (*txt != '\0') { // 在主串中逐个匹配子串
const char *t = txt;
const char *p = pat;
while (*t != '\0' && *p != '\0' && *t == *p) {
t++;
p++;
}
if (*p == '\0') { // 若子串已经匹配完成,则返回子串在主串中首次出现的起始地址
return (char*)txt;
}
txt++; // 否则继续在主串中查找下一次匹配的位置
}
return NULL; // 若主串中没有找到子串,则返回 NULL
}
```
该函数首先判断子串是否为空串,如果是,则返回 NULL。然后在主串中逐个匹配子串,如果匹配成功,则返回子串在主串中首次出现的起始地址,否则继续在主串中查找下一次匹配的位置。如果在主串中没有找到子串,则返回 NULL。
使用示例:
```c
#include <stdio.h>
#include <string.h>
char* StrStr(const char *txt, const char *pat);
int main() {
char m[1024], s[1024], *p;
gets(m);
gets(s);
p = StrStr(m, s);
if (p) {
printf("%d\n", p - m);
} else {
puts("NULL");
}
return 0;
}
```
阅读全文
相关推荐
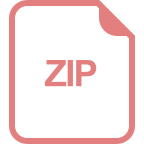
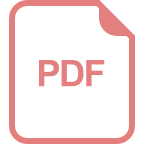

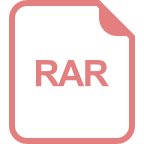
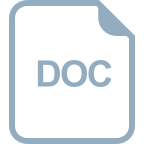
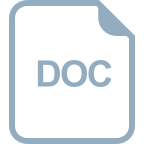

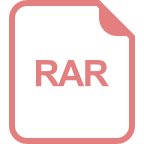
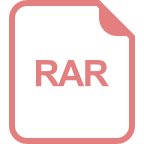
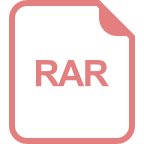
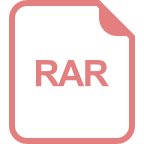
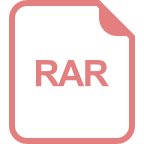





