#include <stdio.h> int main(){ char input; int letter=0,space=0,digit=0,other=0; printf("请输入一行字符:\n"); while ((input=getchar())!='\n'){ if((input>='a'&&input<='z')||(input>='A'&&input<='Z')){ letter++; }else if(input==''){ space++; }else if(input>='0'&&input<='9'){ digit++; }else{ other++; } } printf("其中英文字母有%d个,空格有%d个,数字有%d个,其他字符有%d个。\n",letter,space,digit,other); return 0; }这段代码中有什么错误
时间: 2023-11-28 17:47:41 浏览: 40
这段代码中存在一些错误:
1. 第6行的 `input==''` 应该改为 `input==' '`, 表示检测输入是否为空格。
2. 第8行应该加上大括号 `{}`,表示 if 语句的执行语句块。
修改后的代码如下:
```c
#include <stdio.h>
int main() {
char input;
int letter = 0, space = 0, digit = 0, other = 0;
printf("请输入一行字符:\n");
while ((input = getchar()) != '\n') {
if ((input >= 'a' && input <= 'z') || (input >= 'A' && input <= 'Z')) {
letter++;
} else if (input == ' ') {
space++;
} else if (input >= '0' && input <= '9') {
digit++;
} else {
other++;
}
}
printf("其中英文字母有%d个,空格有%d个,数字有%d个,其他字符有%d个。\n", letter, space, digit, other);
return 0;
}
```
相关问题
c输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数
好的,这是一个比较基础的C语言问题,下面是代码实现:
```c
#include<stdio.h>
#include<ctype.h>
int main() {
char c;
int letter=0, space=0, digit=0, other=0;
printf("Please input a string: \n");
while ((c = getchar()) != '\n') {
if (isalpha(c))
letter++;
else if (isspace(c))
space++;
else if (isdigit(c))
digit++;
else
other++;
}
printf("There are %d letters, %d spaces, %d digits and %d other characters in the string.\n", letter, space, digit, other);
return 0;
}
```
代码中使用了 getchar() 函数从键盘输入一行字符,使用 isalpha()、isspace() 和 isdigit() 函数判断字符的类型,并统计个数,最后输出结果。
@要gpt号请找管理 输入一行字符,分别统计出其中的英文字母、空格、数字和其他字符的个数。求c语言中while语句代码
下面是统计字符个数的C语言代码,使用了while循环和ASCII码:
```c
#include <stdio.h>
int main() {
char input;
int letter = 0, space = 0, digit = 0, other = 0;
printf("请输入一行字符:\n");
while ((input = getchar()) != '\n') {
if ((input >= 'a' && input <= 'z') || (input >= 'A' && input <= 'Z')) {
letter++;
} else if (input == ' ') {
space++;
} else if (input >= '0' && input <= '9') {
digit++;
} else {
other++;
}
}
printf("其中英文字母有%d个,空格有%d个,数字有%d个,其他字符有%d个。\n", letter, space, digit, other);
return 0;
}
```
代码中使用了getchar()函数逐个读取输入的字符,如果是英文字母、空格或数字,则对应变量加1;否则,其他字符个数加1。最后输出各个字符的个数。
相关推荐
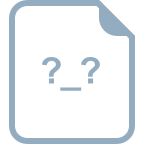
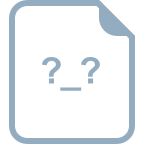
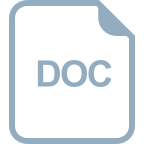
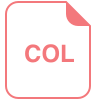
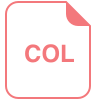







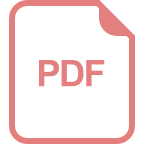
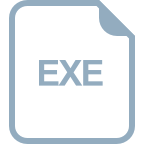
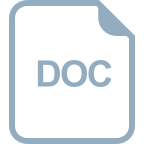
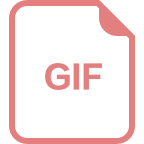