linux C共享内存的方式实现两个线程的示例代码
时间: 2024-02-09 07:10:27 浏览: 28
下面是一个简单的示例代码,演示了如何使用共享内存来实现两个线程的数据交换。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <pthread.h>
#include <sys/shm.h>
#include <sys/ipc.h>
#define SHM_SIZE 1024
int shmid;
void *shmptr;
void *producer(void *arg)
{
char *str = "hello, world";
int len = strlen(str) + 1;
// 将数据写入共享内存
memcpy(shmptr, str, len);
printf("producer: write data to shared memory\n");
return NULL;
}
void *consumer(void *arg)
{
char buf[SHM_SIZE];
// 从共享内存中读取数据
memcpy(buf, shmptr, SHM_SIZE);
printf("consumer: read data from shared memory: %s\n", buf);
return NULL;
}
int main()
{
pthread_t tid1, tid2;
// 创建共享内存
shmid = shmget(IPC_PRIVATE, SHM_SIZE, IPC_CREAT | 0666);
if (shmid == -1) {
perror("shmget");
exit(EXIT_FAILURE);
}
// 映射共享内存到进程地址空间
shmptr = shmat(shmid, NULL, 0);
if (shmptr == (void *) -1) {
perror("shmat");
exit(EXIT_FAILURE);
}
// 创建两个线程,一个生产者,一个消费者
pthread_create(&tid1, NULL, producer, NULL);
pthread_create(&tid2, NULL, consumer, NULL);
// 等待两个线程结束
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
// 删除共享内存
if (shmdt(shmptr) == -1) {
perror("shmdt");
exit(EXIT_FAILURE);
}
if (shmctl(shmid, IPC_RMID, NULL) == -1) {
perror("shmctl");
exit(EXIT_FAILURE);
}
return 0;
}
```
在这个示例中,我们使用shmget函数创建了一个大小为1024字节的共享内存,并使用shmat函数将共享内存映射到进程地址空间中。然后创建了两个线程,一个生产者线程,一个消费者线程。生产者线程将数据写入共享内存,消费者线程从共享内存中读取数据。最后,我们使用shmdt和shmctl函数分别将共享内存从进程地址空间中解除映射,并删除共享内存。
相关推荐
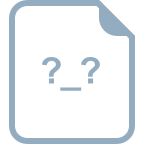
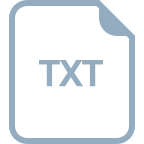
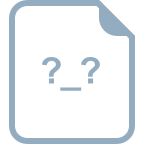














