springboot登录
时间: 2023-08-27 13:07:08 浏览: 81
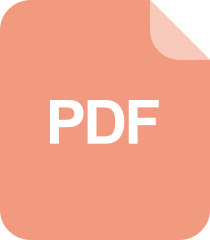
SpringBoot实现登录注册常见问题解决方案
Spring Boot 提供了很多安全特性,可以非常方便地实现用户登录功能。下面是一个简单的 Spring Boot 登录的示例:
1. 配置 Spring Security
在 Spring Boot 项目中,我们可以很方便地使用 Spring Security 来实现安全认证。在 pom.xml 文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
然后在项目中添加一个配置类,用于配置 Spring Security:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/login").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.defaultSuccessUrl("/home")
.permitAll()
.and()
.logout()
.permitAll();
}
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService);
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
这个配置类中,我们通过 @EnableWebSecurity 注解开启了 Spring Security。然后在 configure() 方法中,我们配置了登录页面、成功跳转页面、退出登录等相关信息。在 configureGlobal() 方法中,我们配置了自定义的 UserDetailsService。
2. 实现 UserDetailsService
接下来,我们需要实现 UserDetailsService 接口,用于从数据库或其他数据源中加载用户信息。在这个示例中,我们简单地实现了一个 InMemoryUserDetailsManager,用于存储用户信息。在实际项目中,我们可以从数据库或其他数据源中加载用户信息。
```
@Service
public class UserDetailsServiceImpl implements UserDetailsService {
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
if ("admin".equals(username)) {
List<GrantedAuthority> authorities = new ArrayList<>();
authorities.add(new SimpleGrantedAuthority("ROLE_ADMIN"));
return new User("admin", passwordEncoder().encode("admin"), authorities);
} else {
throw new UsernameNotFoundException("User not found.");
}
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
在这个示例中,我们定义了一个叫做 UserDetailsServiceImpl 的服务类,实现了 UserDetailsService 接口。在 loadUserByUsername() 方法中,我们根据用户名返回了一个 User 对象,其中包含了用户名、密码和角色信息。在这个示例中,我们只定义了一个管理员角色。
3. 实现登录页面
最后,我们需要实现一个登录页面。在这个示例中,我们使用 Thymeleaf 模板引擎实现了一个简单的登录页面:
```
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<form th:action="@{/login}" method="post">
<label>Username: </label><input type="text" name="username" /><br />
<label>Password: </label><input type="password" name="password" /><br />
<input type="submit" value="Sign In" />
</form>
</body>
</html>
```
在这个页面中,我们定义了一个表单,用于输入用户名和密码。表单的 action 属性指向 /login,这个路径与我们在 SecurityConfig 中配置的登录路径一致。在输入用户名和密码后,用户可以通过提交表单来实现登录。
总结
通过以上的步骤,我们实现了一个简单的 Spring Boot 登录功能。在实际项目中,我们可以根据需要进行修改和扩展。
阅读全文
相关推荐
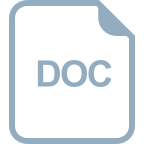
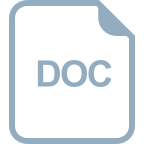
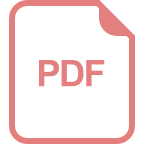
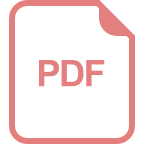
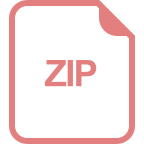
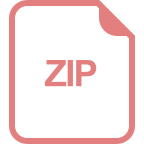
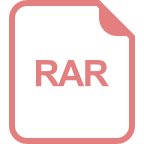
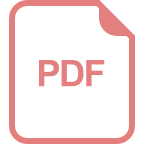
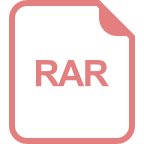
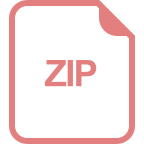
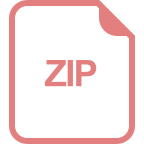
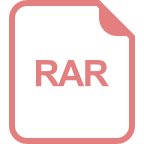
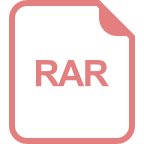
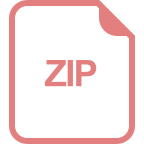
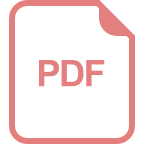
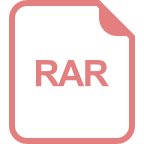
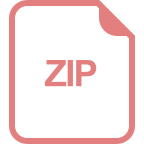