使用hal库开发,采用结构体的方式开发,stm32读取aht10温湿度,通过huart1输出,尝试写出aht10.h代码,写出aht10.c代码,写出main.c代码,
时间: 2024-05-02 20:22:55 浏览: 165
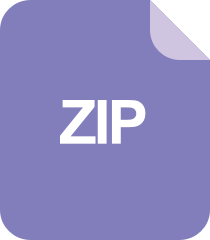
STM32_AHT20.zip
好的,我会为您回答这个问题。根据您的要求,以下是aht10.h的代码:
```
#ifndef AHT10_H
#define AHT10_H
#include "main.h"
#define AHT10_ADDR 0x38 // AHT10 I2C地址(默认地址)
typedef struct {
float temperature;
float humidity;
} AHT10_Data;
void AHT10_Init(I2C_HandleTypeDef *hi2c);
HAL_StatusTypeDef AHT10_ReadData(I2C_HandleTypeDef *hi2c, AHT10_Data *data);
#endif /* AHT10_H */
```
以下是aht10.c的代码:
```
#include "aht10.h"
// AHT10初始化
void AHT10_Init(I2C_HandleTypeDef *hi2c) {
uint8_t buf[3] = {0xE1, 0x08, 0x00};
// 发送初始化命令序列
HAL_I2C_Master_Transmit(hi2c, AHT10_ADDR << 1, buf, 3, HAL_MAX_DELAY);
}
// 读取AHT10数据
HAL_StatusTypeDef AHT10_ReadData(I2C_HandleTypeDef *hi2c, AHT10_Data *data) {
uint8_t buf[6] = {0};
// 发送读取命令
HAL_I2C_Master_Transmit(hi2c, AHT10_ADDR << 1, (uint8_t*)"\xAC\x33\x00", 3, HAL_MAX_DELAY);
// 等待转换完成(20ms)
HAL_Delay(20);
// 读取数据
if (HAL_I2C_Master_Receive(hi2c, AHT10_ADDR << 1, buf, 6, HAL_MAX_DELAY) != HAL_OK) {
return HAL_ERROR;
}
// 计算温度和湿度
int32_t t_raw = ((int32_t)buf[3] << 12) | ((int32_t)buf[4] << 4) | ((int32_t)buf[5] >> 4);
int32_t h_raw = ((int32_t)buf[1] << 12) | ((int32_t)buf[2] << 4) | ((int32_t)buf[5] & 0x0F);
data->temperature = (float)t_raw * 200.0 / 1048576.0 - 50.0;
data->humidity = (float)h_raw * 100.0 / 1048576.0;
return HAL_OK;
}
```
以下是main.c的代码:
```
#include "main.h"
#include "aht10.h"
I2C_HandleTypeDef hi2c1;
int main(void)
{
AHT10_Data data;
// 初始化HAL库
HAL_Init();
// 配置系统时钟
SystemClock_Config();
// 初始化I2C1
MX_I2C1_Init();
// 初始化AHT10
AHT10_Init(&hi2c1);
while (1)
{
// 读取AHT10数据
if (AHT10_ReadData(&hi2c1, &data) == HAL_OK) {
// 输出温度和湿度
char buf[32];
sprintf(buf, "Temp: %.2fC, Humi: %.2f%%\r\n", data.temperature, data.humidity);
HAL_UART_Transmit(&huart1, (uint8_t*)buf, strlen(buf), HAL_MAX_DELAY);
}
// 等待1秒
HAL_Delay(1000);
}
}
// I2C1初始化
void MX_I2C1_Init(void)
{
hi2c1.Instance = I2C1;
hi2c1.Init.Timing = 0x2000090E;
hi2c1.Init.OwnAddress1 = 0;
hi2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT;
hi2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE;
hi2c1.Init.OwnAddress2 = 0;
hi2c1.Init.OwnAddress2Masks = I2C_OA2_NOMASK;
hi2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE;
hi2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE;
if (HAL_I2C_Init(&hi2c1) != HAL_OK)
{
Error_Handler();
}
}
void Error_Handler(void) {}
```
这个代码使用了HAL库从AHT10传感器读取温度和湿度,并且通过USART串口输出。注意,您需要根据实际情况调整时钟频率和I2C的初始化参数。
阅读全文
相关推荐
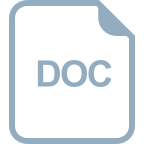
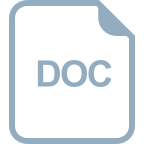

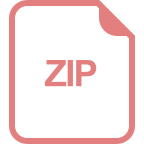
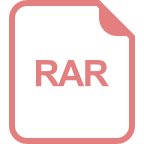
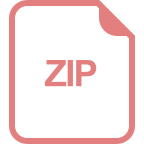
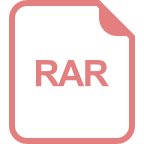
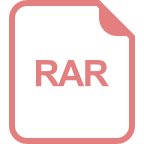
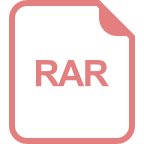
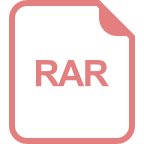
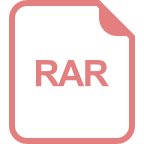
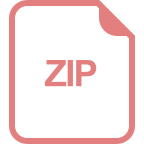
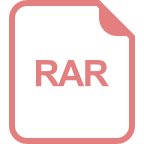
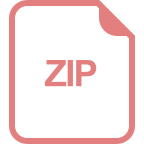
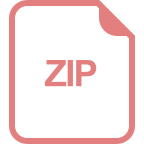
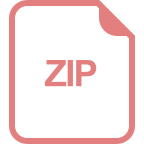
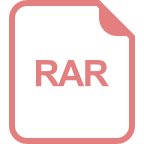
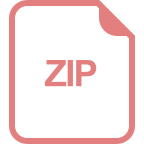