用nodejs做小米商城购物车 实现购物车的增删改查
时间: 2024-01-06 20:03:48 浏览: 27
好的,下面是实现购物车的增删改查的Node.js代码:
首先,我们需要安装相关的依赖包,包括`express`、`body-parser`和`cors`:
```
npm install express body-parser cors --save
```
然后,我们可以创建一个名为`app.js`的文件,编写以下代码:
```javascript
const express = require('express');
const bodyParser = require('body-parser');
const cors = require('cors');
const app = express();
// 使用body-parser中间件解析post请求参数
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
// 使用cors解决跨域问题
app.use(cors());
// 模拟购物车数据
let cartItems = [
{ id: 1, name: '小米手机', price: 1999, quantity: 2 },
{ id: 2, name: '小米电视', price: 2999, quantity: 1 },
];
// 获取购物车数据
app.get('/cart', (req, res) => {
res.json(cartItems);
});
// 添加购物车商品
app.post('/cart', (req, res) => {
const { id, name, price, quantity } = req.body;
const item = cartItems.find(item => item.id === id);
if (item) {
// 如果购物车中已存在该商品,则增加数量
item.quantity += quantity;
} else {
// 否则添加新商品
cartItems.push({ id, name, price, quantity });
}
res.json(cartItems);
});
// 修改购物车商品数量
app.put('/cart/:id', (req, res) => {
const id = parseInt(req.params.id);
const quantity = parseInt(req.body.quantity);
const item = cartItems.find(item => item.id === id);
if (item) {
item.quantity = quantity;
}
res.json(cartItems);
});
// 删除购物车商品
app.delete('/cart/:id', (req, res) => {
const id = parseInt(req.params.id);
cartItems = cartItems.filter(item => item.id !== id);
res.json(cartItems);
});
// 启动服务器
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
```
上面的代码中,我们使用了`express`框架来创建一个简单的服务器,使用了`body-parser`中间件来解析post请求参数,使用了`cors`中间件来解决跨域问题。
我们模拟了一个购物车数据,包括`id`、`name`、`price`和`quantity`四个属性。我们实现了获取购物车数据、添加购物车商品、修改购物车商品数量和删除购物车商品四个接口。
我们可以使用Postman等工具来测试这些接口。例如,我们可以发送一个post请求来添加一个商品:
```
POST http://localhost:3000/cart
{"id": 3, "name": "小米手环", "price": 199, "quantity": 1}
```
然后,我们可以发送一个get请求来获取购物车数据:
```
GET http://localhost:3000/cart
```
会返回如下数据:
```
[
{ "id": 1, "name": "小米手机", "price": 1999, "quantity": 2 },
{ "id": 2, "name": "小米电视", "price": 2999, "quantity": 1 },
{ "id": 3, "name": "小米手环", "price": 199, "quantity": 1 }
]
```
我们还可以发送一个put请求来修改购物车商品数量:
```
PUT http://localhost:3000/cart/1
{"quantity": 3}
```
最后,我们可以发送一个delete请求来删除购物车商品:
```
DELETE http://localhost:3000/cart/3
```
以上就是使用Node.js实现购物车的增删改查的代码。
相关推荐
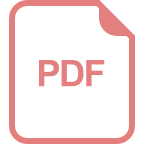
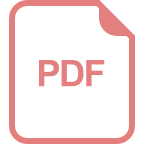














