学生的记录由学号和成绩组成,N名学生的数据已在主函数中放入a结构体数组中,编写一个函数fun,它的功能是:找出成绩最低的学生记录,通过形参返回主函数(规定只有一个最低分)C语言
时间: 2024-09-17 16:01:48 浏览: 53
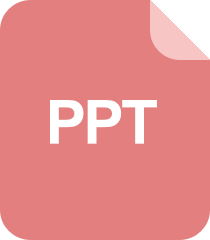
结构体数组的应用-C语言入门教程(1)
在C语言中,你可以创建一个名为`fun`的函数,用于找到学生记录数组中成绩最低的那个。首先,你需要定义一个包含学号和成绩的结构体,例如`student`,然后定义一个辅助函数来比较两个学生的成绩。接下来是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
// 定义学生结构体
typedef struct {
int id; // 学号
int score; // 成绩
} student;
// 比较函数,用于找出较低的成绩
int compare_scores(const void* a, const void* b) {
const student* sa = (const student*) a;
const student* sb = (const student*) b;
return sa->score - sb->score;
}
// 查找最低成绩的函数
student find_lowest_score(student arr[], int n) {
// 使用qsort对数组排序
qsort(arr, n, sizeof(student), compare_scores);
// 返回最后一个元素,即最低成绩的学生
return arr[n - 1];
}
// 主函数
int main() {
student students[N]; // N名学生数据已填充在此数组中
int lowest_id, lowest_score;
// 调用fun函数并获取结果
lowest_score = find_lowest_score(students, N);
lowest_id = lowest_score.id; // 获取学号
printf("最低分数的学生信息: ID %d, Score %d\n", lowest_id, lowest_score.score);
return 0;
}
```
在这个程序中,`compare_scores`函数用于`qsort`排序,它将数组按成绩从低到高排列。`find_lowest_score`函数接受学生数组和数组长度作为输入,最后返回最低分的学生记录。
阅读全文
相关推荐
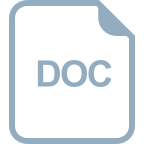
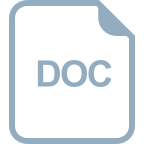














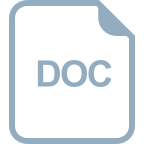
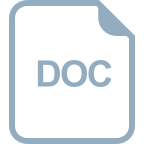