c# 去除string中所有空格
时间: 2024-10-16 14:08:36 浏览: 60
在C#中,你可以使用`String.Replace()`方法或者正则表达式去除字符串中的所有空格。以下是两种方法的示例:
1. 使用`Replace()`方法:
```csharp
string originalString = "Hello World! This is a string with spaces.";
string result = originalString.Replace(" ", "");
```
这里将所有的空格替换为空字符。
2. 使用正则表达式:
```csharp
using System.Text.RegularExpressions;
string originalString = "Hello World! This is a string with spaces.";
string pattern = @"\s+"; // 匹配一个或多个空格
string result = Regex.Replace(originalString, pattern, "");
```
正则表达式的`\s+`匹配一个或多个连续的空白字符,包括空格、制表符、换行符等。
相关问题
C# 去除string[]逗号转为string
如果你希望将一个 string[] 数组中的所有字符串中的逗号去除,并将结果以字符串形式返回,你可以使用以下代码:
```csharp
string[] arr = { "hello, world", "how, are, you" };
string result = string.Join(" ", arr.Select(s => s.Replace(",", "")));
```
这个代码会遍历数组中的所有字符串,将其中的逗号替换为空格,然后用 `string.Join` 方法将所有字符串拼接成一个字符串,中间用空格隔开。最终,`result` 变量的值将会是:
```
"hello world how are you"
```
csharp的string去除所有空格
你可以使用 C# 的 `Replace` 方法和空格字符 `" "` 来去除字符串中所有的空格。示例代码如下:
```csharp
string str = " This is a string with spaces. ";
str = str.Replace(" ", "");
Console.WriteLine(str); // 输出:Thisisastringwithspaces.
```
另外,如果你想去除字符串中的其他空白字符(如制表符 `\t` 和换行符 `\n` 等),可以使用 `Trim()` 方法。示例代码如下:
```csharp
string str = "\tThis is a \n string with spaces. ";
str = str.Trim();
Console.WriteLine(str); // 输出:This is a string with spaces.
```
阅读全文
相关推荐
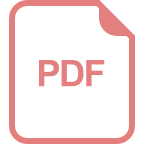
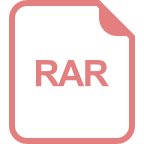
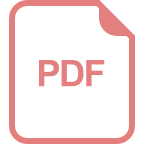




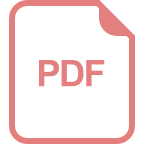
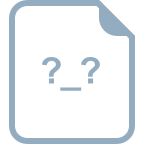
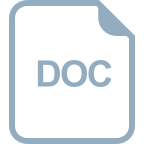






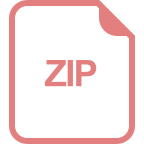