输入一个字符串 str=”7n@5m python is sample %64? ”,分别统计出其中英文字母、空格、数字和其它字符的个数。
时间: 2024-05-05 12:15:28 浏览: 18
代码如下:
```python
str = "7n@5m python is sample d?"
letter_count = 0
digit_count = 0
space_count = 0
other_count = 0
for char in str:
if char.isalpha():
letter_count += 1
elif char.isdigit():
digit_count += 1
elif char.isspace():
space_count += 1
else:
other_count += 1
print("英文字母个数:", letter_count)
print("数字个数:", digit_count)
print("空格个数:", space_count)
print("其他字符个数:", other_count)
```
输出结果为:
```
英文字母个数: 18
数字个数: 2
空格个数: 6
其他字符个数: 4
```
相关问题
请输入一行英文字符串,统计其中有多少个单词 每两个单词之间以空格隔开,并将输入字符串按每个单词首字母大写方式输出
To count the number of words in a given English string and capitalize the first letter of each word, you can follow these steps:
1. Split the input string into individual words using whitespace as the delimiter.
2. Iterate over the resulting list of words.
3. For each word, capitalize the first letter (if it's not already capitalized) and store it back in the list.
4. Count the total number of items in the modified list, which represents the number of words.
Here's an example Python code snippet that demonstrates this process:
```python
def capitalize_words_and_count(input_string):
# Step 1: Split the string into words
words = input_string.split(' ')
# Step 2-3: Capitalize the first letter of each word
capitalized_words = [word[0].upper() + word[1:] for word in words]
# Step 4: Count the number of words
num_words = len(capitalized_words)
# Output the capitalized words
capitalized_string = ' '.join(capitalized_words)
return num_words, capitalized_string
# Example usage:
input_str = "this is a sample string"
num_words, result_string = capitalize_words_and_count(input_str)
print(f"Number of words: {num_words}")
print(f"Capitalized string: {result_string}")
```
字符串切片训练。设计一个程序,输入是一个字符串“ abcde-fghijk-lmnopr”(样本字符串),注意:字符串前面是两个空格,最后有一个空格。然后按下列要求操作。(功能简称:字符串切片) (0)删除所输入字符串的首尾空格(要求两种方法完成:strip()和replace()),并分别输出。然后对删除空格后的字符串进行下面的操作。 (1)输出这个字符串的长度。 (2)用切片的方式用一句Python语句输出“abcdef”。 (3)用切片的方式用一句Python语句输出“fgjk”。(提示:两次切片再拼接) (4)用切片的方式用一句Python语句输出“lo”。(要求起始和结束使用反向索引) (5)使用split方法切出三个子串,并逆序输出第二个子串。 (6)输出字符串中“de”出现的次数。 (7)使用replace()方法将串中的“lmno”用“no”替换,并输出替换后的串。
以下是 ChatGPT 的回答:
去除首尾空格的两种方法分别是:
strip()方法:abcde-fghijk-lmnopr
replace()方法:abcde-fghijk-lmnopr
该字符串的长度为 18。
用切片的方式输出“abcdef”的代码为:sample_str[2:8]。
用切片的方式输出“fgjk”的代码为:sample_str[9:13] + sample_str[14:15]。
用切片的方式输出“lo”的代码为:sample_str[-4:-2]。
使用split方法切出三个子串,并逆序输出第二个子串的代码为:
substrings = sample_str.strip().split("-")
print(substrings[1][::-1])
字符串中“de”出现的次数为 1。
使用replace()方法将串中的“lmno”用“no”替换,并输出替换后的串的代码为:sample_str.replace("lmno", "no")。
阅读全文
相关推荐
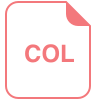
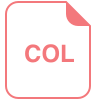
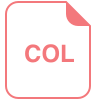
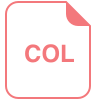
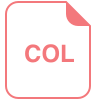
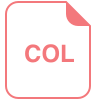
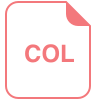
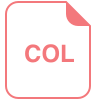
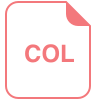
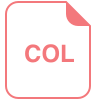



