doc.Root.Element引用using
时间: 2024-11-13 10:18:56 浏览: 9
在.NET的XML文档处理中,`doc.Root.Element` 是 LINQ to XML 模块提供的一个常见操作,它用于访问 XML 文档的根元素。当你有一个 `XDocument` 或 `XElement` 对象 `doc`,`Root` 属性返回了文档的第一个(也是根)元素。`Element` 方法则进一步用于查找指定名称的子元素,如果存在,则返回该元素;如果没有找到匹配的元素,会返回 `null`。
例如:
```csharp
XDocument doc = XDocument.Load("path_to_your_xml_file.xml");
XElement rootElement = doc.Root;
XElement elementToFind = rootElement.Element("YourElementName"); // 如果存在名为 "YourElementName" 的元素,这里将获取该元素
```
如果使用 `Element()` 方法找不到匹配的元素,你可以通过异常处理来检查 `elementToFind` 是否为 `null`:
```csharp
if (elementToFind != null)
{
// Element found and you can work with it
}
else
{
Console.WriteLine("Element not found.");
}
```
相关问题
TinyXMl2用函数实现功能: 获取/设置指定Element下的Attribute的值
在tinyxml2中,您可以使用`Attribute`和`SetAttribute`函数来获取和设置指定元素下的属性值。以下是一个示例代码:
```cpp
#include <iostream>
#include <tinyxml2.h>
using namespace tinyxml2;
int main() {
XMLDocument doc;
doc.LoadFile("example.xml"); // 加载XML文件
// 获取根节点
XMLElement* root = doc.FirstChildElement("root");
if (root == nullptr) {
std::cerr << "Failed to load root element." << std::endl;
return 1;
}
// 获取指定元素下的属性值
XMLElement* element = root->FirstChildElement("child");
if (element != nullptr) {
const char* attributeValue = element->Attribute("attribute");
if (attributeValue != nullptr) {
std::cout << "Attribute value: " << attributeValue << std::endl;
} else {
std::cerr << "Failed to get attribute value." << std::endl;
return 1;
}
} else {
std::cerr << "Failed to find child element." << std::endl;
return 1;
}
// 设置指定元素下的属性值
element->SetAttribute("attribute", "new_value");
// 保存修改后的XML文件
doc.SaveFile("example.xml");
return 0;
}
```
在上述代码中,我们首先使用`LoadFile`函数加载XML文件。然后,我们使用`FirstChildElement`函数获取根节点。接下来,我们使用`FirstChildElement`函数获取指定元素(例如"child")。然后,我们使用`Attribute`函数获取该元素的属性值,并将其打印出来。如果要设置属性值,您可以使用`SetAttribute`函数来更新属性值。最后,我们使用`SaveFile`函数保存修改后的XML文件。
请确保您已经安装了tinyxml2库,并将其包含在您的项目中。您还需要将示例代码中的`example.xml`替换为您要操作的实际XML文件的路径。
希望对您有所帮助!如有任何疑问,请随时提问。
'lxml.etree._ElementUnicodeResult' object has no attribute 'xpath'
This error message indicates that you are trying to use the `xpath` method on an object of the `lxml.etree._ElementUnicodeResult` class, which does not have an `xpath` method.
To fix this error, you need to make sure that you are calling the `xpath` method on an object of the `lxml.etree._Element` class, which is the class that has the `xpath` method.
For example, if you are trying to extract data from an XML document using the `xpath` method, you need to first parse the XML document using the `lxml.etree.parse` method, and then call the `xpath` method on the parsed XML document.
Here is an example code snippet that shows how to do this:
```python
import lxml.etree as ET
# Parse the XML document
doc = ET.parse('example.xml')
# Get the root element
root = doc.getroot()
# Use the xpath method to extract data from the XML document
data = root.xpath('//some/element/path')
# Print the extracted data
print(data)
```
In this example, we first parse the XML document using the `ET.parse` method, and then get the root element of the parsed document using the `doc.getroot` method. We then use the `xpath` method on the root element to extract data from the XML document. Finally, we print the extracted data.
阅读全文
相关推荐
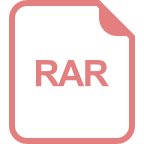
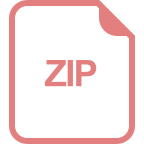
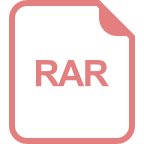
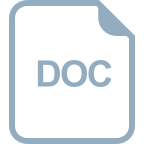
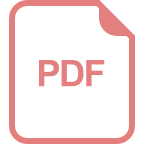
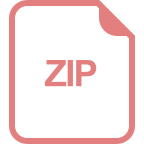
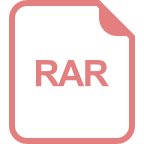
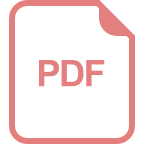
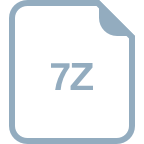
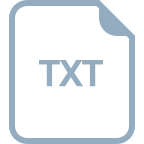
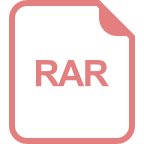
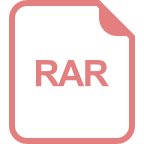
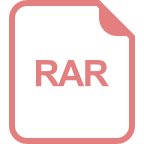
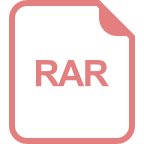
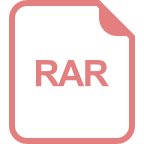
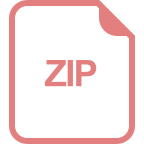

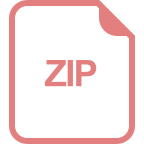