springboot jasypt加密解密配置
时间: 2024-08-17 09:01:22 浏览: 66
Spring Boot集成Jasypt用于安全地存储和处理敏感数据是一种常见的做法。以下是基本的配置步骤:
1. 添加依赖:首先,在你的`pom.xml`或`build.gradle`文件中添加Jasypt的Spring Boot starter依赖。
```xml
<!-- Maven -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jasypt</artifactId>
</dependency>
// Gradle
implementation 'org.springframework.boot:spring-boot-starter-jasypt'
```
2. 配置属性:在`application.properties`或`application.yml`文件中设置相关的加密和解密参数。例如:
```properties
# 加密算法
spring.jasypt.encryptor.algorithm=BCrypt
# 密钥
spring.jasypt.encryptor.password=your_secret_key
# 如果使用环境变量替换硬编码的密钥
spring.jasypt.encryptor.key-alias=jasypt
# 明文源路径或资源名
spring.jasypt.encryptor.encrypt-context-headers=**/*.txt
```
3. 使用@Encryptable注解:如果你想对实体类字段进行加密,可以使用`@Encryptable`注解,并提供一个解密后的getter方法。
```java
import org.springframework.core.annotation.Encryptable;
@Entity
public class User {
@Encryptable
private String password;
//...
}
```
4. 解密服务:如果需要在Controller或Service中进行解密操作,你可以创建一个`PasswordEncoder`实现或使用Spring提供的默认实现:
```java
import org.springframework.context.annotation.Bean;
import org.springframework.stereotype.Component;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
@Component
public class CustomPasswordEncoder implements PasswordEncoder {
//... 实现PasswordEncoder接口的方法
}
```
5. 使用密码编码器:在处理用户注册或登录时,使用自定义的`PasswordEncoder`解码明文密码。
```java
@Autowired
private CustomPasswordEncoder passwordEncoder;
@PostMapping("/register")
public User register(@RequestBody User user) {
user.setPassword(passwordEncoder.encode(user.getPassword()));
return userService.save(user);
}
```
阅读全文
相关推荐
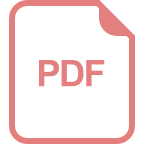
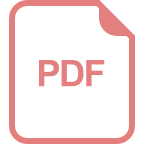
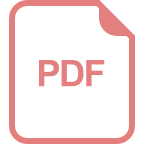




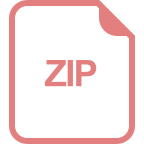
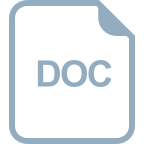
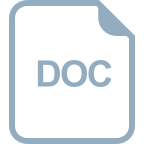
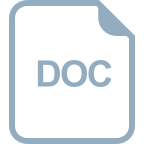
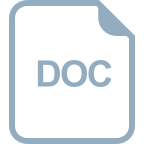
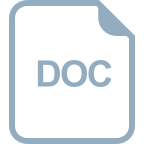




