编写一个Java 程序,使用冒泡排序算法对一个整数数组进行排序,并打印排序前后 的数组。
时间: 2024-09-27 22:03:44 浏览: 32
在Java中,你可以使用冒泡排序算法来对整数数组进行排序。这是一个简单的示例程序:
```java
public class BubbleSortExample {
public static void main(String[] args) {
int[] array = {5, 2, 8, 1, 9}; // 待排序的整数数组
// 打印原始数组
System.out.println("Original Array:");
for (int num : array) {
System.out.print(num + " ");
}
System.out.println();
// 冒泡排序
bubbleSort(array);
// 打印排序后的数组
System.out.println("Sorted Array:");
for (int num : array) {
System.out.print(num + " ");
}
}
// 冒泡排序函数
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// 交换元素
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
}
```
当你运行这个程序,它首先会显示原始数组,然后通过冒泡排序算法对其进行排序,最后打印出排序后的数组。
阅读全文
相关推荐
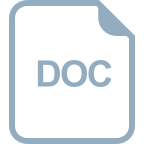
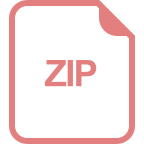
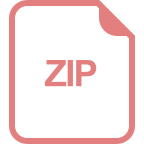















