java 输入年和月份,打印天数 格式为2000年2月有29天
时间: 2023-09-09 09:02:19 浏览: 151
首先,我们可以通过Scanner类获取用户输入的年份和月份。然后,我们需要判断输入的年份是否为闰年,因为闰年的二月有29天。根据闰年的规则,如果年份能够被4整除但不能被100整除,或者能够被400整除,那么就是闰年。
接下来,我们需要判断月份的天数。对于1、3、5、7、8、10、12月份,它们每个月都有31天;对于4、6、9、11月份,它们每个月都有30天;对于2月份,如果是闰年,则有29天,否则有28天。
最后,我们将得到的结果进行输出。
以下是代码示例:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入年份:");
int year = scanner.nextInt();
System.out.print("请输入月份:");
int month = scanner.nextInt();
boolean isLeapYear = (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
String monthString;
int days;
if (month == 1) {
monthString = "一月";
days = 31;
} else if (month == 2) {
monthString = "二月";
if (isLeapYear) {
days = 29;
} else {
days = 28;
}
} else if (month == 3) {
monthString = "三月";
days = 31;
} else if (month == 4) {
monthString = "四月";
days = 30;
} else if (month == 5) {
monthString = "五月";
days = 31;
} else if (month == 6) {
monthString = "六月";
days = 30;
} else if (month == 7) {
monthString = "七月";
days = 31;
} else if (month == 8) {
monthString = "八月";
days = 31;
} else if (month == 9) {
monthString = "九月";
days = 30;
} else if (month == 10) {
monthString = "十月";
days = 31;
} else if (month == 11) {
monthString = "十一月";
days = 30;
} else if (month == 12) {
monthString = "十二月";
days = 31;
} else {
monthString = "";
days = 0;
}
if (monthString.isEmpty()) {
System.out.println("请输入正确的月份!");
} else {
System.out.println(year + "年" + monthString + "有" + days + "天。");
}
}
}
```
希望我的回答对您有帮助!
阅读全文
相关推荐




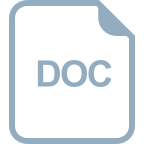











