C#怎么以指定的用户名和密码,向一个Windows网络共享路径中读写文件
时间: 2024-09-27 15:17:58 浏览: 44
在C#中,如果需要使用特定的用户名和密码访问Windows网络共享资源,通常会涉及到NTLM或Kerberos身份验证。但为了简单起见,我们通常不会直接在文件系统操作中提供用户名和密码,而是利用内置的`NetworkCredential`类配合`WebClient`或`System.Net.Sockets`来做安全的网络请求。下面是一个基本的例子,展示如何使用`WebClient`来读写文件,但请注意这并不是推荐的最佳实践,因为这可能导致明文传输敏感信息:
```csharp
using System;
using System.Net;
using System.IO;
public class NetworkShareHandler
{
private readonly string _username;
private readonly string _password;
private readonly string _sharedPath;
public NetworkShareHandler(string username, string password, string sharedPath)
{
_username = username;
_password = password;
_sharedPath = sharedPath;
}
private WebClient GetAuthenticatedWebClient()
{
var client = new WebClient();
client.Credentials = new NetworkCredential(_username, _password);
return client;
}
// 读取文件
public byte[] ReadFile(string fileName)
{
var url = $"http://{_sharedPath}/{fileName}";
using (var client = GetAuthenticatedWebClient())
{
return client.DownloadData(url);
}
}
// 写入文件
public void UploadFile(string fileName, byte[] data)
{
var url = $"http://{_sharedPath}/{fileName}";
using (var client = GetAuthenticatedWebClient())
{
client.UploadData(url, data);
}
}
}
// 使用示例
string username = "your_username";
string password = "your_password";
string sharedPath = "\\192.168.130.32\cyg";
NetworkShareHandler handler = new NetworkShareHandler(username, password, sharedPath);
byte[] fileContent = handler.ReadFile("example.txt"); // 获取文件内容
handler.UploadFile("new_file.txt", fileContent); // 将新内容写入文件
阅读全文
相关推荐

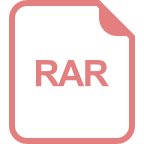






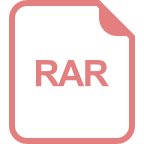









