fasterrcnn可视化
时间: 2023-08-16 16:04:49 浏览: 94
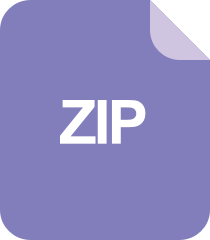
faster rcnn
您可以使用以下步骤将Faster R-CNN模型的预测结果可视化:
1. 导入必要的库和模块:
```python
import torch
import torchvision.transforms as T
from torchvision.models.detection.faster_rcnn import FastRCNNPredictor
from PIL import Image
import matplotlib.pyplot as plt
```
2. 加载训练好的Faster R-CNN模型:
```python
model = torch.load('path_to_model.pth')
model.eval()
```
3. 定义图像预处理函数:
```python
def preprocess_image(image_path):
image = Image.open(image_path).convert('RGB')
transform = T.Compose([T.ToTensor()])
image = transform(image)
return image
```
4. 运行模型并得到预测结果:
```python
image_path = 'path_to_image.jpg'
image = preprocess_image(image_path)
preds = model([image])
```
5. 可视化预测结果:
```python
image = Image.open(image_path).convert('RGB')
plt.imshow(image)
boxes = preds[0]['boxes'].detach().cpu().numpy()
labels = preds[0]['labels'].detach().cpu().numpy()
scores = preds[0]['scores'].detach().cpu().numpy()
for box, label, score in zip(boxes, labels, scores):
if score > 0.5: # 设置一个阈值,只显示置信度大于0.5的物体
x1, y1, x2, y2 = box
plt.rectangle((x1, y1), (x2, y2), color='red', linewidth=2)
plt.text(x1, y1, f'{label}', color='red')
plt.text(x1, y2, f'{score}', color='red')
plt.axis('off')
plt.show()
```
请确保将`path_to_model.pth`替换为您训练好的模型的路径,将`path_to_image.jpg`替换为您要进行预测和可视化的图像路径。
这样,您就可以通过这段代码来可视化Faster R-CNN模型的预测结果了。希望能对您有所帮助!
阅读全文
相关推荐
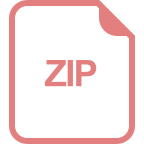


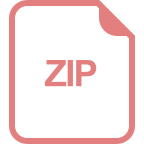
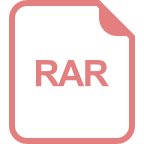
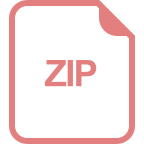
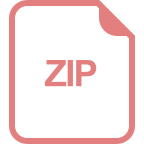
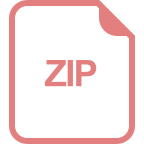
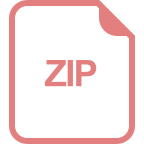
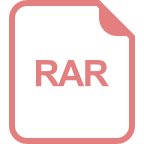
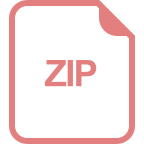
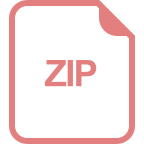
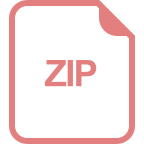
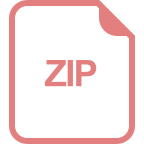
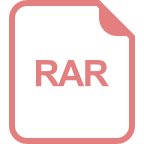
