根据以上描述生成一段完整的c语言代码
时间: 2024-12-19 21:13:51 浏览: 4
基于上述描述,这里是一个简单的C语言代码片段,展示了如何使用A*算法在一个8x8的数组中解决八数码问题。请注意,这只是一个简化版的基本框架,实际应用可能需要更复杂的错误处理和数据结构。
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
// 棋盘格结构体,包含坐标和值
typedef struct Node {
int row, col;
int value;
} Node;
// A*函数原型
Node* a_star_search(int start_row, int start_col, int goal_row, int goal_col, Node grid[8][8]);
// ...其他辅助函数(如邻接节点查找、估价函数等)
// 主函数
int main() {
int grid[8][8] = {...}; // 初始化棋盘格
int start_row = ...; // 起点行
int start_col = ...; // 起点列
int goal_row = ...; // 目标行
int goal_col = ...; // 目标列
Node *solution = a_star_search(start_row, start_col, goal_row, goal_col, grid);
if (solution != NULL) {
printf("Solution found: ");
while (solution != NULL) {
printf("(%d,%d) Value: %d ", solution->row, solution->col, solution->value);
solution = solution->next;
}
printf("\n");
} else {
printf("No solution found.\n");
}
return 0;
}
// A*函数实现
Node* a_star_search(int start_row, int start_col, int goal_row, int goal_col, Node grid[8][8]) {
// ...实际的A*算法核心代码
}
```
在这个代码中,`a_star_search`函数负责整个搜索过程,而`main`函数则是程序入口,负责设置初始棋盘和目标位置,并调用`a_star_search`。具体的实现细节,如邻接节点查找、启发式函数计算(例如Manhattan距离)以及数据结构(链表表示路径)等,需要根据实际需求进一步编写。
阅读全文
相关推荐
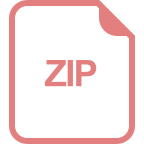
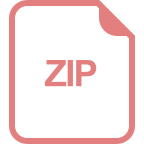
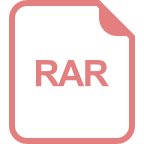

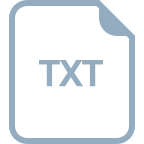
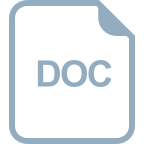
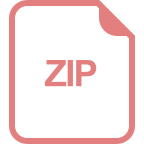
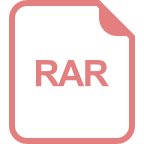
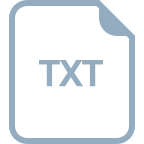
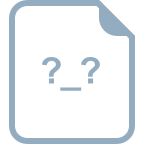
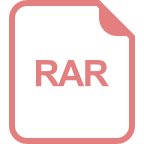
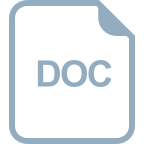
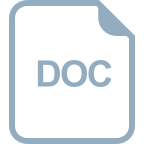
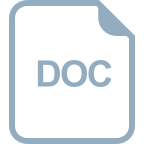
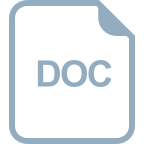
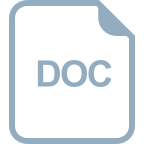
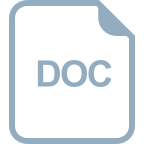

