编写C程序,使用Linux操作系统中的信号量机制模拟解决经典的进程同步问题:生产者-消费问题。假设有一个生产者和消费者,缓冲区可以存放产品,生产者不断生产产品放入缓冲区,消费者不断从缓冲区中取出产品并消费。
时间: 2024-12-13 10:14:45 浏览: 4
在C程序中,我们可以使用Linux的信号量(semaphores)来管理生产者-消费者问题。信号量是一种同步原语,它维护了一个计数,用于控制对共享资源的操作。以下是简化的示例:
首先,我们需要包括必要的头文件,并定义三个关键的数据结构:生产者、消费者和信号量。
```c
#include <stdio.h>
#include <stdlib.h>
#include <semaphore.h>
typedef struct {
int products; // 缓冲区的产品数量
sem_t full; // 生产者信号量,当满时等待
sem_t empty; // 消费者信号量,当空时等待
} ProdCons;
```
接下来,我们为生产者和消费者创建函数,分别负责生产和消费产品:
```c
void producer(ProdCons *pc, int limit) {
while (1) {
sem_wait(&pc->full); // 当缓冲区满时,生产者等待
pc->products++;
printf("Producer produced a product, count now %d\n", pc->products);
sem_post(&pc->empty); // 通知一个空位给消费者
}
}
void consumer(ProdCons *pc, int limit) {
while (1) {
sem_wait(&pc->empty); // 当缓冲区空时,消费者等待
if (pc->products == 0) {
printf("No more products, exiting...\n");
break;
}
pc->products--;
printf("Consumer consumed a product, count now %d\n", pc->products);
sem_post(&pc->full); // 通知生产者缓冲区有空间了
}
}
```
最后,在主函数中初始化信号量并启动生产者和消费者线程:
```c
int main() {
ProdCons pc = {0, SEM_INIT(1, 1), SEM_INIT(1, 1)}; // 初始化两个信号量
pthread_t producer_thread, consumer_thread;
if (pthread_create(&producer_thread, NULL, producer, &pc)) {
perror("Producer thread creation failed");
return -1;
}
if (pthread_create(&consumer_thread, NULL, consumer, &pc)) {
perror("Consumer thread creation failed");
return -1;
}
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
return 0;
}
```
在这个例子中,`SEM_INIT`用于初始化信号量,`sem_wait`使当前线程阻塞直到信号量计数值大于零,`sem_post`将信号量递减并唤醒等待线程。通过这种方式,生产者和消费者能够安全地共享和操作缓冲区。
阅读全文
相关推荐


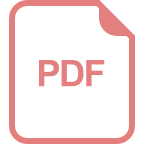
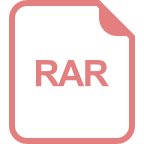
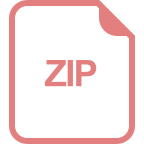
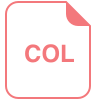
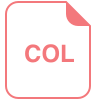
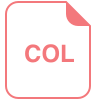
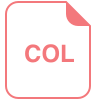
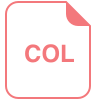
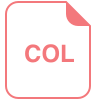
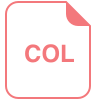
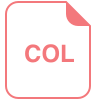
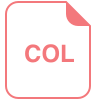
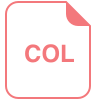
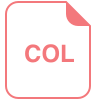
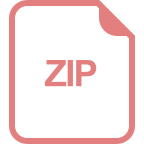