java 双线程实现拷贝2个文件
时间: 2024-10-11 10:09:32 浏览: 43
在 Java 中,你可以通过创建两个独立的线程来并行地复制两个文件。以下是使用 `java.util.concurrent` 包中的 `ExecutorService` 和 `Callable` 接口的一个简单示例:
```java
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.concurrent.*;
public class FileCopyWithThreads {
private static final int BUFFER_SIZE = 4096;
public static void main(String[] args) throws IOException, InterruptedException {
String sourcePath = "sourceFile.txt";
String destinationPath = "destinationFile.txt";
ExecutorService executor = Executors.newFixedThreadPool(2); // 创建固定大小的线程池
CopyTask copyTaskSource = new CopyTask(sourcePath, destinationPath + "_source");
CopyTask copyTaskDestination = new CopyTask(sourcePath, destinationPath);
Future<Void> futureSource = executor.submit(copyTaskSource);
Future<Void> futureDestination = executor.submit(copyTaskDestination);
futureSource.get(); // 等待源文件复制完成
futureDestination.get(); // 等待目标文件复制完成
executor.shutdown(); // 关闭线程池
System.out.println("Both files copied successfully.");
}
static class CopyTask implements Callable<Void> {
private final String sourcePath;
private final String outputPath;
public CopyTask(String sourcePath, String outputPath) {
this.sourcePath = sourcePath;
this.outputPath = outputPath;
}
@Override
public Void call() throws IOException {
Path source = Paths.get(sourcePath);
Path target = Paths.get(outputPath);
try (BufferedInputStream in = Files.newInputStream(source);
BufferedOutputStream out = Files.newOutputStream(target)) {
byte[] buffer = new byte[BUFFER_SIZE];
while (in.read(buffer) != -1) {
out.write(buffer);
}
}
return null;
}
}
}
```
在这个例子中,我们创建了两个 `CopyTask` 类的实例,每个任务负责复制其中一个文件。然后将它们提交给线程池处理。`call()` 方法实现了实际的文件复制操作。
阅读全文
相关推荐










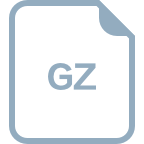


