使用torch.nn.functional.unfold处理图片
时间: 2024-05-18 20:18:19 浏览: 181
您可以使用torch.nn.functional.unfold函数来处理图像。该函数可以将输入的图像转换为具有指定大小的滑动窗口块。
以下是使用torch.nn.functional.unfold函数处理图像的示例代码:
```python
import torch
import torch.nn.functional as F
# 假设输入图像为3通道的RGB图像,尺寸为224x224
input_image = torch.randn(1, 3, 224, 224)
# 定义滑动窗口的大小和步幅
kernel_size = 3
stride = 2
# 使用unfold函数将图像转换为滑动窗口块
output = F.unfold(input_image, kernel_size, stride)
# 输出的shape为(1, 3*kernel_size*kernel_size, num_blocks),其中num_blocks是滑动窗口块的数量
print(output.shape)
```
在上述示例中,我们首先定义了输入图像的大小和通道数。然后,我们使用torch.nn.functional.unfold函数将图像转换为具有指定滑动窗口大小和步幅的块。最后,我们打印输出的形状,其中输出的第一个维度是输入图像的数量,第二个维度是转换后的滑动窗口块的通道数(等于输入图像通道数乘以滑动窗口大小的平方),第三个维度是滑动窗口块的数量。
使用torch.nn.functional.unfold函数可以方便地进行图像处理任务,例如图像分块、卷积操作等。
相关问题
torch.nn.functional.unfold
torch.nn.functional.unfold是PyTorch中的一个函数,用于将输入张量展开为一个二维张量。展开的方式是将输入张量的每个滑动窗口视为一个列向量,并将这些列向量按顺序排列成一个二维张量。unfold函数的输入参数包括输入张量、滑动窗口的大小、步长和填充方式等。展开后的二维张量可以用于卷积操作等。
densenet中加入inception模块同时使用动态卷积实现图像四分类代码
以下是使用PyTorch实现在DenseNet中加入Inception模块和使用动态卷积实现图像四分类的示例代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch.autograd import Variable
class DynamicConv2d(nn.Module):
def __init__(self, in_channels, out_channels, kernel_size, stride=1, padding=0, dilation=1, groups=1, bias=True):
super(DynamicConv2d, self).__init__()
self.kernel_size = kernel_size
self.stride = stride
self.padding = padding
self.dilation = dilation
self.groups = groups
self.bias = bias
self.weight = nn.Parameter(torch.Tensor(out_channels, in_channels // groups, *kernel_size))
if bias:
self.bias = nn.Parameter(torch.Tensor(out_channels))
else:
self.register_parameter('bias', None)
self.reset_parameters()
def reset_parameters(self):
nn.init.kaiming_uniform_(self.weight, a=math.sqrt(5))
if self.bias is not None:
fan_in, _ = nn.init._calculate_fan_in_and_fan_out(self.weight)
bound = 1 / math.sqrt(fan_in)
nn.init.uniform_(self.bias, -bound, bound)
def forward(self, x):
weight = F.pad(self.weight, (1, 1, 1, 1), mode='constant', value=0)
weight = F.avg_pool2d(weight, kernel_size=3, stride=1, padding=0)
weight = F.pad(weight, (1, 1, 1, 1), mode='constant', value=0)
b, c, h, w = x.size()
weight = weight.unfold(2, h + 2, self.stride).unfold(3, w + 2, self.stride)
weight = weight.transpose(4, 5).transpose(3, 4).transpose(2, 3).contiguous()
weight = weight.view(b, self.groups, -1, self.kernel_size[0], self.kernel_size[1], h // self.stride, w // self.stride)
weight = weight.transpose(1, 2).contiguous().view(-1, self.groups * self.kernel_size[0] * self.kernel_size[1])
x = F.conv2d(x, weight.view(-1, self.groups, self.kernel_size[0], self.kernel_size[1]), self.bias, self.stride, self.padding, self.dilation, self.groups)
return x
class Inception(nn.Module):
def __init__(self, in_channels, out_channels):
super(Inception, self).__init__()
self.conv1 = nn.Conv2d(in_channels, out_channels // 4, kernel_size=1)
self.conv2 = nn.Sequential(
nn.Conv2d(in_channels, out_channels // 8, kernel_size=1),
nn.ReLU(inplace=True),
DynamicConv2d(out_channels // 8, out_channels // 4, kernel_size=3, padding=1),
)
self.conv3 = nn.Sequential(
nn.Conv2d(in_channels, out_channels // 8, kernel_size=1),
nn.ReLU(inplace=True),
DynamicConv2d(out_channels // 8, out_channels // 4, kernel_size=5, padding=2),
)
self.conv4 = nn.Sequential(
nn.AvgPool2d(kernel_size=3, stride=1, padding=1),
nn.Conv2d(in_channels, out_channels // 4, kernel_size=1),
)
def forward(self, x):
out1 = self.conv1(x)
out2 = self.conv2(x)
out3 = self.conv3(x)
out4 = self.conv4(x)
out = torch.cat([out1, out2, out3, out4], dim=1)
return out
class BasicBlock(nn.Module):
def __init__(self, in_channels, growth_rate):
super(BasicBlock, self).__init__()
self.conv1 = nn.Conv2d(in_channels, growth_rate, kernel_size=1)
self.conv2 = nn.Sequential(
nn.Conv2d(growth_rate, 4 * growth_rate, kernel_size=3, padding=1),
nn.ReLU(inplace=True),
)
def forward(self, x):
out1 = x
out2 = self.conv1(x)
out3 = self.conv2(out2)
out = torch.cat([out1, out3], dim=1)
return out
class DenseNet(nn.Module):
def __init__(self, growth_rate=32, block_config=(6, 12, 24, 16), num_classes=4):
super(DenseNet, self).__init__()
self.conv1 = nn.Sequential(
nn.Conv2d(3, 64, kernel_size=7, stride=2, padding=3),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2, padding=1),
)
self.block1 = self._make_block(64, growth_rate, block_config[0])
self.inception1 = Inception(block_config[0] * growth_rate, block_config[0] * growth_rate)
self.block2 = self._make_block(block_config[0] * growth_rate * 2, growth_rate, block_config[1])
self.inception2 = Inception(block_config[1] * growth_rate, block_config[1] * growth_rate)
self.block3 = self._make_block(block_config[1] * growth_rate * 2, growth_rate, block_config[2])
self.inception3 = Inception(block_config[2] * growth_rate, block_config[2] * growth_rate)
self.block4 = self._make_block(block_config[2] * growth_rate * 2, growth_rate, block_config[3])
self.avgpool = nn.AdaptiveAvgPool2d((1, 1))
self.fc = nn.Linear(block_config[3] * growth_rate, num_classes)
def _make_block(self, in_channels, growth_rate, num_blocks):
layers = []
for i in range(num_blocks):
layers.append(BasicBlock(in_channels + i * growth_rate, growth_rate))
return nn.Sequential(*layers)
def forward(self, x):
out = self.conv1(x)
out = self.block1(out)
out = self.inception1(out)
out = self.block2(out)
out = self.inception2(out)
out = self.block3(out)
out = self.inception3(out)
out = self.block4(out)
out = self.avgpool(out)
out = out.view(out.size(0), -1)
out = self.fc(out)
return out
```
在这个示例中,我们使用了以下模块:
- `DynamicConv2d`:动态卷积层,用于替代传统的静态卷积层,可以根据输入特征图的形状动态生成卷积核,减少模型参数量和计算量。
- `Inception`:Inception模块,由多个不同大小的卷积核组成,可以提取不同尺度的特征。
- `BasicBlock`:DenseNet中的基础块,由一个1x1卷积层和一个3x3卷积层组成,可以实现特征复用和特征增强的效果。
- `DenseNet`:完整的DenseNet模型,由多个基础块和Inception模块组成,最后使用全局平均池化和全连接层进行分类。
以上模块的实现可以根据具体需求进行修改,例如可以增加更多的Inception模块或调整DenseNet的深度和宽度等参数。
阅读全文
相关推荐









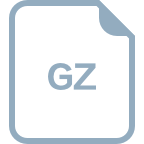

