vue3+ts 怎么封装全局的 防抖和节流函数
时间: 2023-09-01 19:09:00 浏览: 202
在Vue 3中结合TypeScript封装全局的防抖和节流函数可以通过创建一个`utils`文件,并在Vue应用的入口文件中进行全局注册。下面是一个示例:
```typescript
// utils/debounce.ts
export function debounce(func: Function, delay: number) {
let timer: NodeJS.Timeout | null = null;
return function(...args: any[]) {
if (timer) {
clearTimeout(timer);
}
timer = setTimeout(() => {
func.apply(this, args);
}, delay);
};
}
// utils/throttle.ts
export function throttle(func: Function, delay: number) {
let timer: NodeJS.Timeout | null = null;
let lastRun = 0;
return function(...args: any[]) {
const currentTime = Date.now();
if (timer) {
clearTimeout(timer);
}
if (currentTime - lastRun >= delay) {
func.apply(this, args);
lastRun = currentTime;
} else {
timer = setTimeout(() => {
func.apply(this, args);
lastRun = Date.now();
}, delay);
}
};
}
```
然后,在Vue应用的入口文件(如`main.ts`)中,可以将这些函数注册为全局方法:
```typescript
import { createApp } from 'vue';
import App from './App.vue';
import { debounce, throttle } from './utils';
const app = createApp(App);
app.config.globalProperties.$debounce = debounce;
app.config.globalProperties.$throttle = throttle;
app.mount('#app');
```
现在,你可以在Vue组件中使用`this.$debounce`和`this.$throttle`来调用防抖和节流函数了。
```vue
<template>
<div>
<input type="text" @input="handleInput" />
</div>
</template>
<script>
export default {
methods: {
handleInput: this.$debounce(function(event) {
console.log(event.target.value);
}, 300),
},
};
</script>
```
在上面的示例中,`handleInput`方法使用了防抖函数,延迟300毫秒后执行。你可以根据实际需求调整防抖和节流的延迟时间。
阅读全文
相关推荐
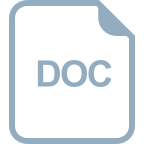
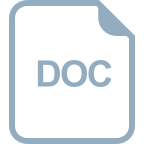
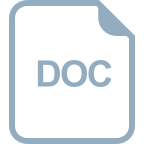
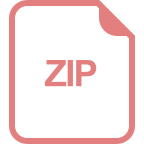
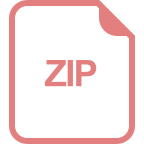
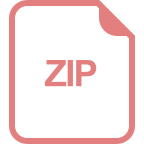
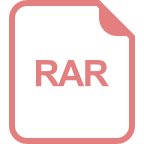
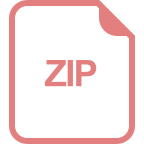
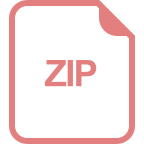
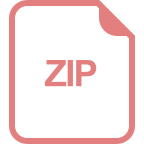
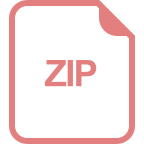
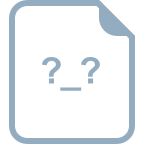
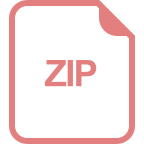
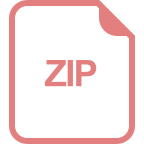
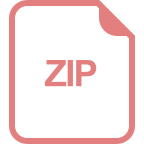
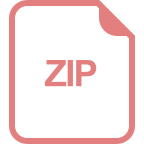
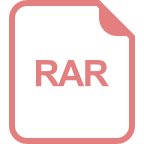
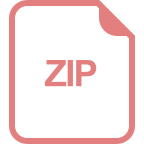