java 选择日期,下载指定日期的文件,纯前端实现,给出具体代码示例
时间: 2024-09-09 13:13:08 浏览: 48
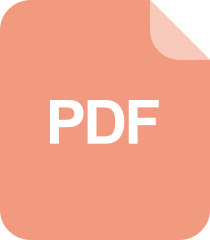
java实现文件上传下载和图片压缩代码示例
在JavaScript中,特别是在前端环境下,我们可以使用HTML的`<input type="date">`元素让用户选择日期,并结合AJAX技术来发送请求下载特定日期的文件。以下是简单的一个前端实现思路:
首先,创建一个HTML表单让用户选择日期:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Download File by Date</title>
</head>
<body>
<label for="selectDate">Select a date:</label>
<input type="date" id="selectDate" name="selectedDate">
<button onclick="downloadFile()">Download</button>
<script src="script.js"></script>
</body>
</html>
```
然后,在`script.js`文件中处理用户选择的日期并下载文件:
```javascript
function downloadFile() {
const selectedDate = document.getElementById('selectDate').value;
const url = `https://example.com/files/${selectedDate}.txt`; // 替换为实际文件URL
// 创建隐藏的form元素用于发起GET请求
const form = new FormData();
form.append("date", selectedDate);
fetch(url, {
method: 'GET',
body: form,
headers: { 'Content-Type': 'application/x-www-form-urlencoded' } // 配置适合的头信息
})
.then(response => {
if (!response.ok) {
throw new Error(`Error! Response code: ${response.status}`);
}
return response.blob(); // 返回响应体作为Blob对象
})
.then(blob => {
// 创建a标签模拟点击下载
const aLink = document.createElement('a');
aLink.href = URL.createObjectURL(blob);
aLink.download = `${selectedDate}.txt`;
aLink.click();
})
.catch(error => console.error(error));
}
```
这个例子假设文件名与选定日期相关,并且服务器接受包含日期参数的GET请求。在实际应用中,你需要根据服务器的实际API和文件命名规则进行调整。
阅读全文
相关推荐
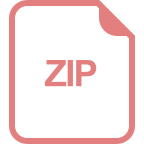
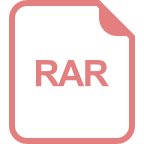
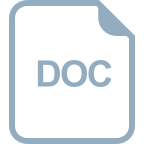
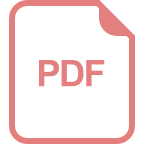
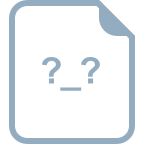
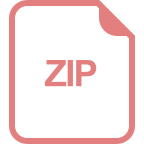
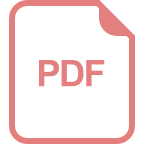
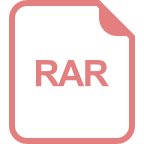
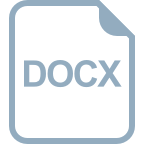
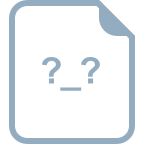
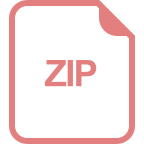
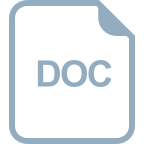
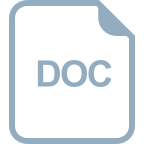
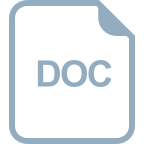
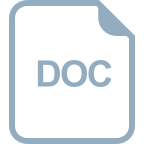
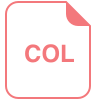
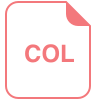

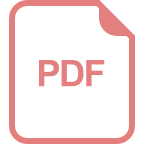