sql 根据指定的文件名找到可执行文件的代码
时间: 2023-09-30 12:00:27 浏览: 134
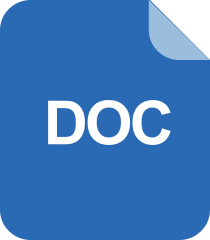
sql文件代码
SQL是一种用于管理和操作关系型数据库的语言,主要用于存储和检索数据。因此,SQL本身不能直接用于查找文件名并找到可执行文件的代码。然而,可以使用编程语言(如Python、Java或C++)结合SQL来完成这个任务。
以下是使用Python编写的示例代码:
```python
import os
import sqlite3
# 连接数据库
connection = sqlite3.connect('file_database.db')
cursor = connection.cursor()
# 创建包含文件名和可执行文件路径的表
cursor.execute('CREATE TABLE IF NOT EXISTS files (filename TEXT PRIMARY KEY, filepath TEXT)')
# 插入样例数据
cursor.execute("INSERT INTO files VALUES ('test.exe', 'C:/path/to/test.exe')")
cursor.execute("INSERT INTO files VALUES ('sample.exe', 'C:/path/to/sample.exe')")
# 可在实际应用中使用其他方式添加数据,如从用户输入或文件读取
# 提交事务并关闭连接
connection.commit()
connection.close()
# 从数据库中获取指定文件名的可执行文件路径
def get_executable_file_path(filename):
connection = sqlite3.connect('file_database.db')
cursor = connection.cursor()
cursor.execute("SELECT filepath FROM files WHERE filename = ?", (filename,))
result = cursor.fetchone()
connection.close()
if result:
return result[0]
else:
return "未找到该文件"
# 测试代码
filename = "test.exe"
executable_file_path = get_executable_file_path(filename)
print(f"文件 {filename} 的可执行文件路径为:{executable_file_path}")
```
上述代码使用`sqlite3`模块创建了一个名为`file_database.db`的SQLite数据库文件,并在其中创建了一个名为`files`的表。该表包含两列,分别用于存储文件名和可执行文件的路径。
在插入样例数据后,通过编写`get_executable_file_path`函数,可以从数据库中查询指定文件名的可执行文件路径。
这只是一个简单的示例,实际应用中可能需要根据具体的需求进行一些额外的处理和优化。
阅读全文
相关推荐
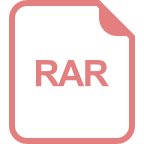
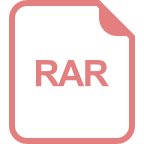
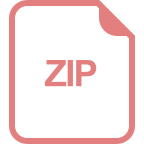
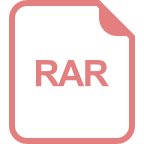
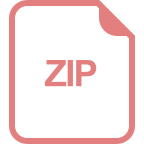
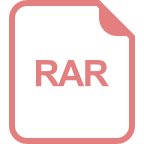
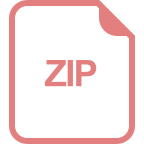
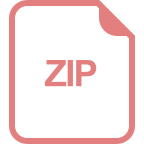
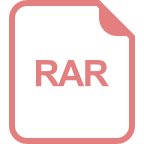
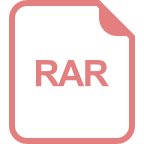
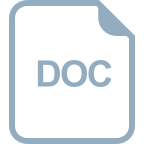
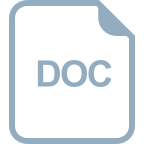
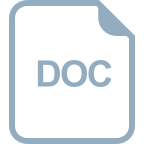
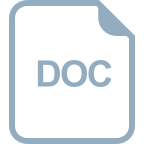
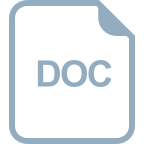