STM32上位机与STM32通讯
时间: 2023-08-17 10:05:38 浏览: 118
可以使用串口通信实现STM32上位机与STM32的通讯。在STM32上位机端,你可以使用串口发送数据给STM32,而STM32则可以通过串口接收这些数据。以下是一个简单的示例代码,演示如何在STM32上位机和STM32之间进行串口通信:
在STM32上位机端:
```c
#include "stm32f4xx.h"
// 配置串口
void USART_Config(void) {
// 使能串口时钟
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART3, ENABLE);
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOB, ENABLE);
// 配置串口引脚
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10 | GPIO_Pin_11;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
// 将引脚映射到串口功能
GPIO_PinAFConfig(GPIOB, GPIO_PinSource10, GPIO_AF_USART3); // USART3_TX
GPIO_PinAFConfig(GPIOB, GPIO_PinSource11, GPIO_AF_USART3); // USART3_RX
// 配置串口参数
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART3, &USART_InitStructure);
// 使能串口
USART_Cmd(USART3, ENABLE);
}
// 发送数据
void USART_SendData(uint8_t data) {
while (USART_GetFlagStatus(USART3, USART_FLAG_TXE) == RESET);
USART_SendData(USART3, data);
}
int main(void) {
// 初始化串口
USART_Config();
while (1) {
// 向STM32发送数据
USART_SendData('A');
delay_ms(1000); // 延时1秒
}
}
```
在STM32端:
```c
#include "stm32f4xx.h"
// 配置串口
void USART_Config(void) {
// 使能串口时钟
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
// 配置串口引脚
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 将引脚映射到串口功能
GPIO_PinAFConfig(GPIOA, GPIO_PinSource2, GPIO_AF_USART2); // USART2_TX
GPIO_PinAFConfig(GPIOA, GPIO_PinSource3, GPIO_AF_USART2); // USART2_RX
// 配置串口参数
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART2, &USART_InitStructure);
// 使能串口
USART_Cmd(USART2, ENABLE);
}
// 接收数据
uint8_t USART_ReceiveData(void) {
while (USART_GetFlagStatus(USART2, USART_FLAG_RXNE) == RESET);
return (uint8_t)USART_ReceiveData(USART2);
}
int main(void) {
// 初始化串口
USART_Config();
while (1) {
// 接收来自上位机的数据
uint8_t data = USART_ReceiveData();
// 处理接收到的数据
// ...
}
}
```
以上代码仅为示例,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
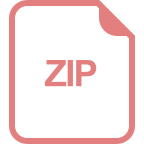
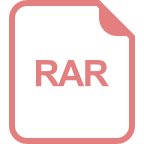
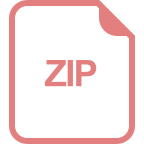
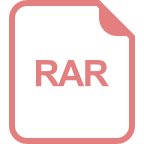
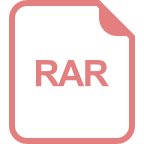
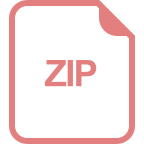
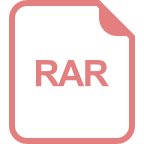
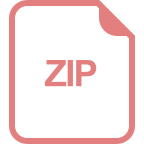
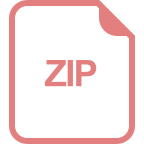
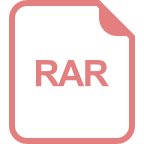
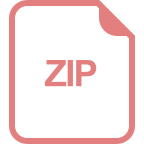
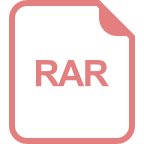
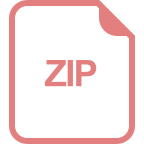
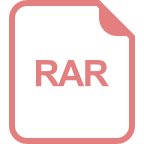
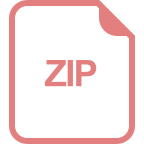
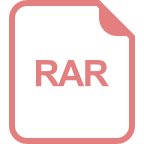
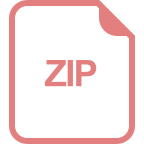