vue前端是通过什么属性和springboot后端接口再到数据库查询数据的。请写一个示范案例:前端是用户名和密码 后端写一个校验用户名和密码的接口。让它们实现联调。假设后端地址是8.148.25.76
时间: 2024-10-07 08:01:42 浏览: 25
在Vue前端和SpringBoot后端的联调中,通常会利用HTTP请求库(如axios)来进行数据交互。前端需要设置一个表单来收集用户输入的用户名和密码,并通过API发送POST请求到后端服务器进行验证。以下是一个简单的示例:
**Vue组件示例 (index.vue)**
```html
<template>
<div>
<form @submit.prevent="login">
<input v-model="username" placeholder="请输入用户名" type="text" />
<input v-model="password" placeholder="请输入密码" type="password" />
<button type="submit">登录</button>
</form>
<p v-if="message">{{ message }}</p> <!-- 显示错误消息 -->
</div>
</template>
<script>
export default {
data() {
return {
username: '',
password: '',
message: ''
};
},
methods: {
login() {
this.$http.post('http://8.148.25.76/auth/login', { username: this.username, password: this.password })
.then(response => {
if (response.data.success) {
// 登录成功处理
} else {
this.message = response.data.error;
}
})
.catch(error => {
console.log('登录失败:', error);
this.message = '网络错误或登录信息有误';
});
}
}
};
// 假设使用vue-resource或axios这样的库
// 如果使用axios,需安装`npm install axios`
// 如果使用vue-resource,则在main.js中引入并配置
</script>
```
**SpringBoot后端控制层 (LoginController.java)**
```java
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class LoginController {
@PostMapping("/auth/login")
public Result login(@RequestBody LoginRequest request) {
String actualUsername = request.getUsername();
String actualPassword = request.getPassword();
// 这里模拟数据库校验逻辑
boolean isValid = checkAuthentication(actualUsername, actualPassword);
if (isValid) {
return new Result(true, "登录成功");
} else {
return new Result(false, "用户名或密码错误");
}
}
private boolean checkAuthentication(String username, String password) {
// ... 实现实际的验证逻辑,比如查询数据库等
}
}
// 示例模型类
class LoginRequest {
private String username;
private String password;
// getters and setters
}
```
在这个例子中,当用户点击登录按钮时,Vue前端会将用户名和密码封装成JSON格式的`LoginRequest`对象,并通过POST请求发送到指定的SpringBoot后端接口。后端接收到请求后检查提供的用户名和密码是否匹配预期逻辑,如果验证通过则返回成功响应,否则返回错误信息。
阅读全文
相关推荐
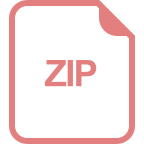
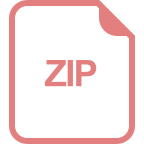
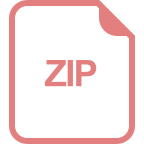















