yyyy-mm-dd hh:MM转为时间戳
时间: 2024-09-28 12:07:39 浏览: 11
"yyyy-MM-dd HH:mm" 格式的日期和时间转换成Unix时间戳(自1970年1月1日 00:00:00 GMT起至现在的秒数或毫秒数),通常涉及到将日期字符串解析成日期对象,然后将其转换为时间戳。在JavaScript中,可以使用`Date`对象配合`getTime()`方法来完成这个过程,示例如下:
```javascript
function dateToTimestamp(dateStr) {
// 解析字符串到日期对象
var date = new Date(dateStr);
// 转换为时间戳(单位为毫秒)
var timestamp = date.getTime();
return timestamp;
}
// 使用函数转换 "2023-04-05 12:30:00" 到时间戳
var dateString = "2023-04-05 12:30:00";
var timestamp = dateToTimestamp(dateString);
console.log(timestamp); // 输出对应的时间戳数值
```
如果你需要的是秒级时间戳,可以直接除以1000。
相关问题
yyyy-MM-dd HH:mm:ss 转为时间戳
时间戳是指从1970年1月1日00:00:00 UTC(协调世界时)开始计算的秒数。要将yyyy-MM-dd HH:mm:ss格式的时间转换为时间戳,您可以使用编程语言提供的日期时间函数或库。
以下是一个示例,假设您使用Python编程语言:
```python
import datetime
def convert_to_timestamp(date_string):
date_format = "%Y-%m-%d %H:%M:%S"
datetime_obj = datetime.datetime.strptime(date_string, date_format)
timestamp = datetime_obj.timestamp()
return int(timestamp)
date_string = "yyyy-MM-dd HH:mm:ss"
timestamp = convert_to_timestamp(date_string)
print(timestamp)
```
请将"yyyy-MM-dd HH:mm:ss"替换为您要转换的实际日期时间字符串。运行此代码将打印出相应的时间戳。
yyyy-MM-dd HH:mm:ss 获取当前时间戳
在Java中,如果你想获取当前日期和时间并按照"yyyy-MM-dd HH:mm:ss"这种格式展示为字符串,你可以使用Java 8引入的LocalDateTime类配合DateTimeFormatter进行格式化。首先,你需要创建一个LocalDateTime对象表示当前时间,然后使用DateTimeFormatter将其转换为指定的字符串格式。以下是示例代码:
```java
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class Main {
public static void main(String[] args) {
LocalDateTime now = LocalDateTime.now(); // 获取当前时间
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"); // 设置日期时间格式
String formattedDate = now.format(formatter); // 格式化为字符串
System.out.println(formattedDate);
}
}
```
运行这段代码,你会得到类似"2023-04-06 13:45:00"这样的当前时间戳。
相关推荐
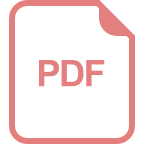
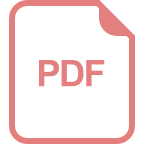
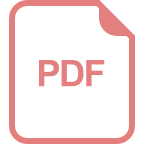












