输入一个字符串,判断字符串中的元素是否全为数字?全为字母?全大写?全小写?用python写,不能用字符串函数,用for循环和ord()函数
时间: 2024-10-27 07:06:21 浏览: 12
在Python中,我们可以使用`for`循环、`if`条件和`ord()`函数来逐个检查字符串中的字符,然后判断它们是否符合指定的条件。以下是一些示例代码:
1. 判断字符串全由数字组成:
```python
def is_all_digits(s):
for char in s:
if not (48 <= ord(char) <= 57): # ASCII码值65-90代表大写字母,97-122代表小写字母
return False
return True
# 使用示例
s = "12345"
print(is_all_digits(s)) # 输出:True
```
2. 判断字符串全由字母组成(不分大小写):
```python
def is_all_letters(s):
for char in s:
if not (65 <= ord(char) <= 90 or 97 <= ord(char) <= 122): # 区分大小写则使用65-90和97-122
return False
return True
# 使用示例
s = "Hello"
print(is_all_letters(s)) # 输出:False
```
3. 判断字符串全为大写字母:
```python
def is_all_uppercase(s):
for char in s:
if ord(char) < 65 or ord(char) > 90: # ASCII码值65代表'A'
return False
return True
# 使用示例
s = "HELLO"
print(is_all_uppercase(s)) # 输出:True
```
4. 判断字符串全为小写字母:
```python
def is_all_lowercase(s):
for char in s:
if ord(char) < 97 or ord(char) > 122: # ASCII码值97代表'a'
return False
return True
# 使用示例
s = "hello"
print(is_all_lowercase(s)) # 输出:True
```
阅读全文
相关推荐
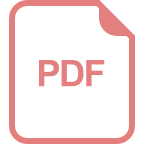
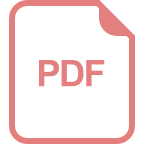
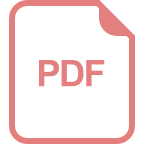






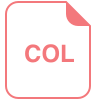
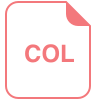








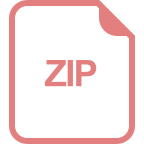