Qt访问两个不同路径下的txt文件,如何将其中一个txt文件的内容加入到另外一个txt文件内容的下面
时间: 2024-09-27 08:02:18 浏览: 47
在Qt中,你可以使用QFile类来读取和操作文件。如果你需要从一个txt文件读取内容,并将其追加到另一个txt文件的末尾,可以按照以下步骤进行:
1. 导入所需的库:
```cpp
#include <QObject>
#include <QFile>
#include <QDebug>
```
2. 创建一个函数来处理这个任务:
```cpp
void appendToFile(const QString &sourceFilePath, const QString &targetFilePath) {
QFile sourceFile(sourceFilePath);
QFile targetFile(targetFilePath);
if (!sourceFile.open(QIODevice::ReadOnly)) {
qDebug() << "Failed to open source file: " + sourceFilePath;
return;
}
if (!targetFile.open(QIODevice::Append | QIODevice::Text)) {
qDebug() << "Failed to open target file: " + targetFilePath;
sourceFile.close();
return;
}
QByteArray sourceContent = sourceFile.readAll();
targetFile.write(sourceContent);
sourceFile.close();
targetFile.close();
}
```
在这个函数中,我们首先打开源文件以只读模式,然后尝试打开目标文件以追加模式。如果打开成功,我们就读取源文件的所有内容,然后将其写入目标文件。最后别忘了关闭两个文件。
3. 调用函数并传递文件路径:
```cpp
QString srcPath = "path_to_first_txt";
QString tgtPath = "path_to_second_txt";
appendToFile(srcPath, tgtPath);
```
替换`"path_to_first_txt"`和`"path_to_second_txt"`为实际的文件路径。
阅读全文
相关推荐
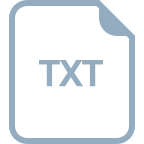
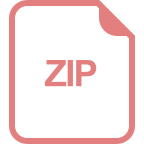
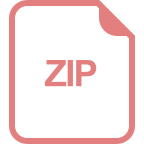
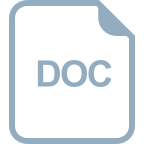
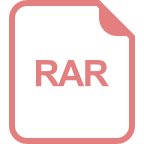
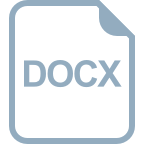
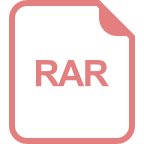
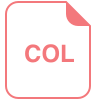
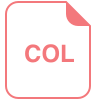
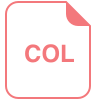
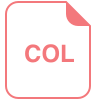
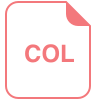
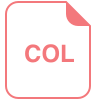
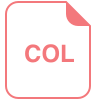
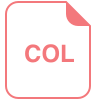
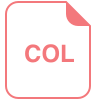
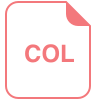
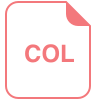