java抽象工厂模式实例
时间: 2023-12-02 17:42:41 浏览: 84
以下是一个Java抽象工厂模式的实例,该实例演示了如何使用抽象工厂模式创建不同操作系统下的按钮和文本框:
```java
// 创建抽象工厂接口
interface GUIFactory {
Button createButton();
TextField createTextField();
}
// 创建具体工厂类 - Windows工厂
class WindowsFactory implements GUIFactory {
public Button createButton() {
return new WindowsButton();
}
public TextField createTextField() {
return new WindowsTextField();
}
}
// 创建具体工厂类 - MacOS工厂
class MacOSFactory implements GUIFactory {
public Button createButton() {
return new MacOSButton();
}
public TextField createTextField() {
return new MacOSTextField();
}
}
// 创建抽象产品接口 - 按钮
interface Button {
void paint();
}
// 创建具体产品类 - Windows按钮
class WindowsButton implements Button {
public void paint() {
System.out.println("Windows Button");
}
}
// 创建具体产品类 - MacOS按钮
class MacOSButton implements Button {
public void paint() {
System.out.println("MacOS Button");
}
}
// 创建抽象产品接口 - 文本框
interface TextField {
void paint();
}
// 创建具体产品类 - Windows文本框
class WindowsTextField implements TextField {
public void paint() {
System.out.println("Windows TextField");
}
}
// 创建具体产品类 - MacOS文本框
class MacOSTextField implements TextField {
public void paint() {
System.out.println("MacOS TextField");
}
}
// 创建客户端类
class Application {
private Button button;
private TextField textField;
public Application(GUIFactory factory) {
button = factory.createButton();
textField = factory.createTextField();
}
public void paint() {
button.paint();
textField.paint();
}
}
// 测试代码
public class Main {
public static void main(String[] args) {
// 创建Windows工厂
GUIFactory factory = new WindowsFactory();
// 创建应用程序
Application app = new Application(factory);
// 绘制UI
app.paint();
// 创建MacOS工厂
factory = new MacOSFactory();
// 创建应用程序
app = new Application(factory);
// 绘制UI
app.paint();
}
}
```
阅读全文
相关推荐
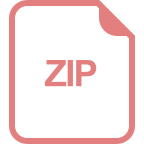
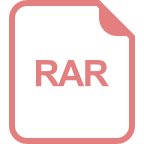
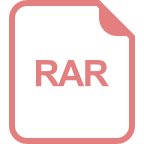
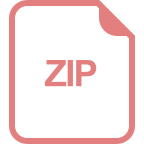
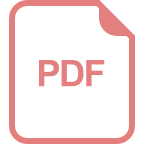
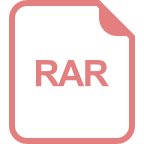
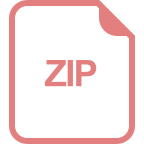
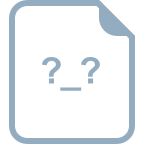
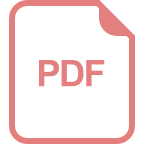
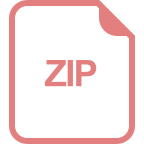
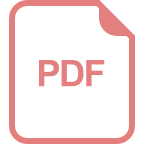
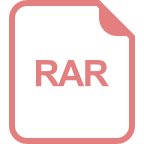
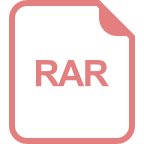
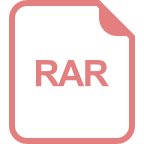
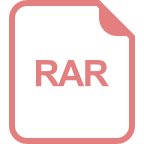
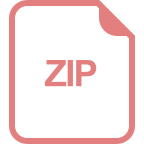