c++小写字符串转大写字符串
时间: 2023-08-12 11:09:38 浏览: 165
你可以使用C++的标准库函数`std::toupper`来将小写字符串转换为大写字符串。下面是一个示例代码:
```cpp
#include <iostream>
#include <cctype> // 包含toupper函数的头文件
int main() {
std::string str = "hello world";
for (char& c : str) {
c = std::toupper(c); // 将每个字符转换为大写
}
std::cout << str << std::endl; // 输出大写字符串
return 0;
}
```
这段代码将字符串"hello world"转换为全大写字符串"HELLO WORLD"并输出。你可以根据自己的需求修改代码中的字符串。
相关问题
c++string字符串大写转小写
可以通过循环遍历字符串并调用 `tolower()` 函数来实现将大写字母转换为小写字母,例如:
```c++
#include <iostream>
#include <string>
int main() {
std::string s = "HELLO WORLD!";
for (char& c : s) {
c = tolower(c);
}
std::cout << s << std::endl; // 输出 "hello world!"
return 0;
}
```
在循环中,将字符串中的每个字符都转换为小写字母,并将其重新赋值给原来的位置。最后输出转换后的字符串即可。
devc++输入一串字符将大写转小写 小写转大写
可以使用头文件`<ctype.h>`中的函数`tolower()`和`toupper()`来实现大写转小写和小写转大写。
示例代码如下:
```c++
#include <iostream>
#include <ctype.h>
using namespace std;
int main() {
string str;
cout << "请输入一串字符:";
getline(cin, str); // 输入一行字符串
// 大写转小写
for (int i = 0; i < str.length(); i++) {
str[i] = tolower(str[i]);
}
cout << "大写转小写后的字符串为:" << str << endl;
// 小写转大写
for (int i = 0; i < str.length(); i++) {
str[i] = toupper(str[i]);
}
cout << "小写转大写后的字符串为:" << str << endl;
return 0;
}
```
输出结果:
```
请输入一串字符:Hello World!
大写转小写后的字符串为:hello world!
小写转大写后的字符串为:HELLO WORLD!
```
相关推荐
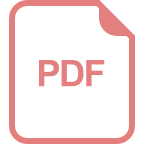
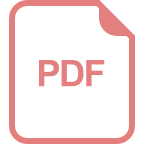












