dataframe series
时间: 2023-11-18 21:50:04 浏览: 81
将DataFrame转换成Series可以通过以下方法实现:
1. 使用DataFrame的`stack()`方法,将DataFrame转换成一个多层级索引的Series。
2. 使用DataFrame的`iloc[]`方法,选择一列数据,并将其转换成Series。
3. 使用DataFrame的`squeeze()`方法,将DataFrame转换成Series。如果DataFrame只有一列数据,则转换后的Series将不再具有列名。
例如,假设有以下DataFrame:
```
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
```
可以使用以上方法将其转换成Series:
```
# 方法一:使用stack()方法
s1 = df.stack()
# 方法二:使用iloc[]方法
s2 = df.iloc[:, ]
# 方法三:使用squeeze()方法
s3 = df.squeeze()
```
相关问题
dataframe series filter
A DataFrame is a two-dimensional table of data, consisting of rows and columns. A Series is a one-dimensional array-like object that can hold any data type, such as integers, strings, or even other objects.
Filtering in a DataFrame or Series involves selecting a subset of data that meets certain conditions. For example, if you have a DataFrame with information about customers, you might want to filter the data to only include customers who live in a certain state.
To filter a DataFrame or Series, you can use boolean indexing. This involves creating a Boolean mask, which is a series of True or False values that indicate whether each element in the DataFrame or Series meets the specified condition.
For example, to filter a DataFrame to only include customers who live in California, you could create a boolean mask like this:
```
mask = customer_data['state'] == 'CA'
```
This will create a Series of True and False values, where True corresponds to customers who live in California and False corresponds to customers who live in other states.
You can then use this mask to filter the DataFrame like this:
```
california_customers = customer_data[mask]
```
This will create a new DataFrame that only includes the rows where the mask is True, which in this case are the customers who live in California.
dataframe series 取值
要从DataFrame或Series中提取值,可以使用以下方法:
1. 使用索引或标签:使用方括号 `[]` 来访问特定列或行的值。例如,对于DataFrame `df`,可以使用 `df['column_name']` 来获取特定列的值,或者使用 `df.loc[row_label]` 来获取特定行的值。
2. 使用位置索引:使用 `.iloc[]` 来按照位置索引获取值。例如,对于DataFrame `df`,可以使用 `df.iloc[row_index, col_index]` 来获取特定位置的值。
3. 使用布尔索引:可以使用布尔条件来过滤DataFrame或Series,并仅返回满足条件的行或元素。例如,对于DataFrame `df`,可以使用 `df[df['column_name'] > value]` 来获取满足条件的行。
下面是一些示例代码:
```python
import pandas as pd
# 创建一个示例DataFrame
data = {'Name': ['John', 'Emma', 'Tom'],
'Age': [25, 28, 22],
'City': ['New York', 'London', 'Paris']}
df = pd.DataFrame(data)
# 使用索引或标签获取特定列的值
name_column = df['Name']
print(name_column)
# 使用索引或标签获取特定行的值
first_row = df.loc[0]
print(first_row)
# 使用位置索引获取特定位置的值
value = df.iloc[1, 2]
print(value)
# 使用布尔索引过滤满足条件的行
filtered_df = df[df['Age'] > 23]
print(filtered_df)
```
这些方法可以根据具体的需求和数据结构进行调整和组合使用。希望对你有帮助!
阅读全文
相关推荐
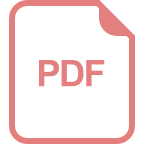














