c++查找字符串在哪行
时间: 2023-12-28 09:03:26 浏览: 69
你可以使用C++的标准库函数`std::string::find`来查找一个字符串在另一个字符串中的位置。然而,C++本身没有提供直接查找字符串在哪一行的功能,因为字符串在内存中是连续存储的,没有明确的行概念。
如果你想查找一个字符串在文件中的行数,你可以按行读取文件,并在每一行中使用`std::string::find`来查找目标字符串。如果找到了目标字符串,则当前行就是你要找的行。
以下是一个示例代码,用于演示如何在文件中查找指定字符串所在的行数:
```cpp
#include <iostream>
#include <fstream>
#include <string>
int findStringInFile(const std::string& filename, const std::string& target) {
std::ifstream inFile(filename);
if (!inFile) {
std::cerr << "Failed to open file: " << filename << std::endl;
return -1;
}
std::string line;
int lineNumber = 0;
while (std::getline(inFile, line)) {
lineNumber++;
if (line.find(target) != std::string::npos) {
inFile.close();
return lineNumber;
}
}
inFile.close();
return -1; // 表示未找到目标字符串
}
int main() {
std::string filename = "example.txt";
std::string target = "hello";
int lineNumber = findStringInFile(filename, target);
if (lineNumber != -1) {
std::cout << "The target string is found in line " << lineNumber << std::endl;
} else {
std::cout << "The target string is not found in the file." << std::endl;
}
return 0;
}
```
请注意,这只适用于普通文本文件,如果需要处理其他类型的文件(如二进制文件),可能需要使用不同的方法。
阅读全文
相关推荐
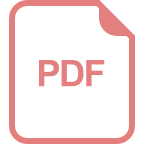
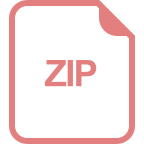
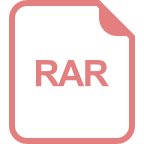
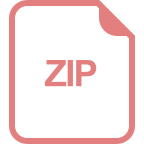
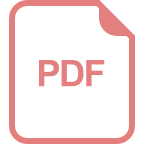
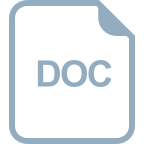
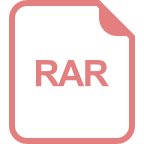
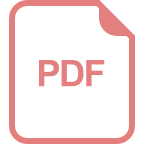
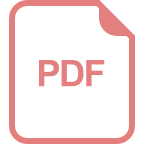
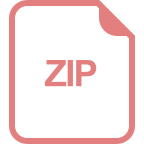
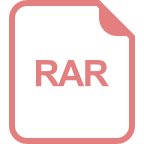
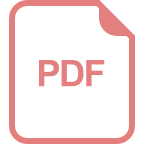
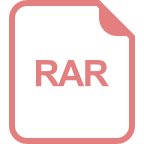
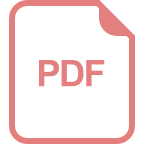
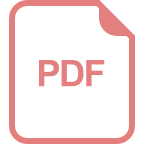
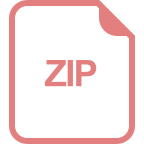
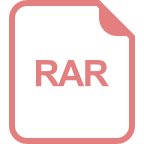