输入一个正整数n。输出n!的最高位上的数字。 输入 输入一个正整数n(n不超过1000)。 输出 输出n!的最高位上的数字。 C语言
时间: 2024-10-19 19:12:24 浏览: 32
在C语言中,计算阶乘并找到最高位数字通常涉及到两个步骤:首先计算阶乘,然后分析结果找出最高位。由于直接计算大数值可能会导致溢出,我们可以采用递归或者循环结合取模的方式来避免这个问题。这里是一个简单的思路:
1. 定义一个函数 `int highestDigit(int n)` 来计算n的阶乘并返回最高位数字。我们从n开始,每次将当前数乘以前面所有数的积,并对10取余,这可以保证结果始终在0到9之间,因为我们只关心最高位。
```c
#include <stdio.h>
// 计算阶乘并记录最高位
int factorialAndDigit(int n, int current_product, int* max_digit) {
if (n == 1 || n == 0) {
// 当n为1或0时,阶乘为1,最高位是1
*max_digit = 1;
return current_product % 10;
}
else {
int new_product = (current_product * n) % 10;
if (new_product > *max_digit) {
*max_digit = new_product; // 更新最高位
}
return factorialAndDigit(n - 1, new_product, max_digit);
}
}
int main() {
int n, highest_digit = 0;
scanf("%d", &n);
if (n <= 1000) {
highest_digit = factorialAndDigit(n, 1, &highest_digit); // 阶乘的起点是1
printf("最高位数字: %d\n", highest_digit);
} else {
printf("抱歉,n超过1000,无法计算。\n");
}
return 0;
}
```
阅读全文
相关推荐














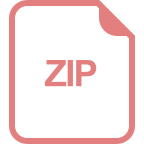
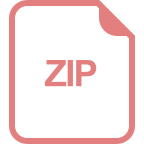
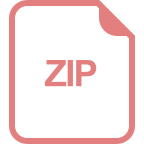
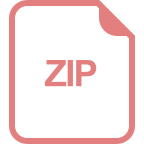
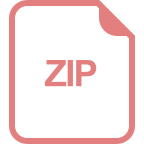